String result = intent.getStringExtra("object"); Log.d(TAG, "json: " + result); JSONObject res = new JSONObject(result); JSONObject object = res.getJSONObject("object"); query = intent.getStringExtra(KEY_RULE_RAW); String api = object.getString("api"); if (res.has("intent")) { String intent = res.getString("intent"); if (intent.equals("addViaPoint")) { // 添加途经点 pi = new PoiSearchInfo(Constant.MAP_SEARCH_CITY, 5000, false, null, object.getString("keyword")); isWayPoint = true; } else if (intent.equals("editViaPoint")) { // 修改途经点 Activity tempActivity = App.getMultiRoundActivity(); if (tempActivity instanceof MapBaseActivity) { ((MapBaseActivity) tempActivity).deleteWayPoint(); } pi = new PoiSearchInfo(Constant.MAP_SEARCH_CITY, 5000, false, null, object.getString("keyword")); isWayPoint = true; } else if (intent.equals("delViaPoint")) { // 删除途经点 Activity tempActivity = App.getMultiRoundActivity(); if (tempActivity instanceof MapBaseActivity) { ((MapBaseActivity) tempActivity).deleteWayPoint(); isWayPoint = false; } } } 以上代码写的怎么样?代码可以优化吗?
时间: 2024-02-02 14:03:17 浏览: 25
这段代码是用来解析一个包含特定格式的 JSON 字符串,根据 JSON 中的字段值来执行不同的操作。代码的整体结构看上去比较清晰,但可以进行一些优化。
首先,这段代码中使用了多个重复的字符串常量,如 "object"、"intent"、"addViaPoint" 等。建议将这些常量定义为静态 final 字段,避免在代码中多次出现,提高代码的可维护性。
其次,代码中使用了多个嵌套的 if-else 语句,增加了代码的复杂度。可以考虑使用 Map 来存储不同的操作对应的处理逻辑,从而避免多层嵌套的 if-else 语句。
最后,建议对代码进行异常处理,例如在解析 JSON 字符串时可能会抛出 JSONException 异常,需要进行捕获和处理。
下面是对代码进行优化的示例:
```
private static final String KEY_OBJECT = "object";
private static final String KEY_INTENT = "intent";
private static final String INTENT_ADD_VIA_POINT = "addViaPoint";
private static final String INTENT_EDIT_VIA_POINT = "editViaPoint";
private static final String INTENT_DEL_VIA_POINT = "delViaPoint";
// 定义不同的操作对应的处理逻辑
private static final Map<String, Consumer<JSONObject>> ACTION_MAP = new HashMap<>();
static {
ACTION_MAP.put(INTENT_ADD_VIA_POINT, object -> {
PoiSearchInfo pi = new PoiSearchInfo(Constant.MAP_SEARCH_CITY, 5000, false, null, object.getString("keyword"));
isWayPoint = true;
});
ACTION_MAP.put(INTENT_EDIT_VIA_POINT, object -> {
Activity tempActivity = App.getMultiRoundActivity();
if (tempActivity instanceof MapBaseActivity) {
((MapBaseActivity) tempActivity).deleteWayPoint();
}
PoiSearchInfo pi = new PoiSearchInfo(Constant.MAP_SEARCH_CITY, 5000, false, null, object.getString("keyword"));
isWayPoint = true;
});
ACTION_MAP.put(INTENT_DEL_VIA_POINT, object -> {
Activity tempActivity = App.getMultiRoundActivity();
if (tempActivity instanceof MapBaseActivity) {
((MapBaseActivity) tempActivity).deleteWayPoint();
isWayPoint = false;
}
});
}
String result = intent.getStringExtra(KEY_OBJECT);
Log.d(TAG, "json: " + result);
JSONObject res = new JSONObject(result);
JSONObject object = res.getJSONObject(KEY_OBJECT);
query = intent.getStringExtra(KEY_RULE_RAW);
String api = object.getString("api");
if (res.has(KEY_INTENT)) {
String intent = res.getString(KEY_INTENT);
Consumer<JSONObject> action = ACTION_MAP.get(intent);
if (action != null) {
action.accept(object);
}
}
```
相关推荐
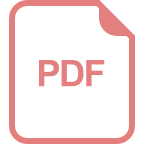
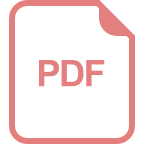
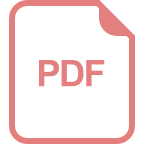











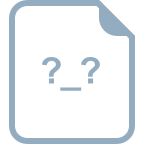
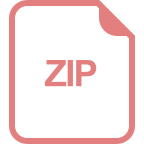
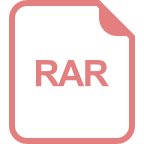