opecv人像左右安人肩膀,上面按头顶剪切
时间: 2023-10-22 21:35:48 浏览: 106
要用OpenCV对人像进行左右安肩膀、上面按头顶剪切,可以按照以下步骤进行:
1. 导入必要的库和模块:cv2、numpy
```python
import cv2
import numpy as np
```
2. 读取原始图片
```python
img = cv2.imread('path/to/image')
```
3. 对图片进行剪切,只保留人像的上半部分
```python
height, width = img.shape[:2]
half_height = int(height / 2)
upper_body_img = img[0:half_height, :]
```
4. 检测人像的关键点
```python
protoFile = "path/to/pose_deploy_linevec.prototxt"
weightsFile = "path/to/pose_iter_440000.caffemodel"
net = cv2.dnn.readNetFromCaffe(protoFile, weightsFile)
keypoints_list = []
threshold = 0.1
inWidth = upper_body_img.shape[1]
inHeight = upper_body_img.shape[0]
net.setInput(cv2.dnn.blobFromImage(upper_body_img, 1.0 / 255, (inWidth, inHeight), (0, 0, 0), swapRB=False, crop=False))
output = net.forward()
H = output.shape[2]
W = output.shape[3]
for i in range(15):
probMap = output[0, i, :, :]
minVal, prob, minLoc, point = cv2.minMaxLoc(probMap)
x = (inWidth * point[0]) / W
y = (inHeight * point[1]) / H
if prob > threshold:
keypoints_list.append((int(x), int(y)))
else:
keypoints_list.append(None)
```
5. 根据关键点来截取人像
```python
left_shoulder = keypoints_list[5]
right_shoulder = keypoints_list[2]
top_head = keypoints_list[0]
if left_shoulder is not None and right_shoulder is not None and top_head is not None:
x1 = left_shoulder[0]
y1 = top_head[1]
x2 = right_shoulder[0]
y2 = y1 + (x2 - x1)
cropped_img = upper_body_img[y1:y2, x1:x2]
```
6. 显示剪切后的图片
```python
cv2.imshow('image', cropped_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
将上述代码整合起来,可以得到以下完整代码:
```python
import cv2
import numpy as np
img = cv2.imread('path/to/image')
height, width = img.shape[:2]
half_height = int(height / 2)
upper_body_img = img[0:half_height, :]
protoFile = "path/to/pose_deploy_linevec.prototxt"
weightsFile = "path/to/pose_iter_440000.caffemodel"
net = cv2.dnn.readNetFromCaffe(protoFile, weightsFile)
keypoints_list = []
threshold = 0.1
inWidth = upper_body_img.shape[1]
inHeight = upper_body_img.shape[0]
net.setInput(cv2.dnn.blobFromImage(upper_body_img, 1.0 / 255, (inWidth, inHeight), (0, 0, 0), swapRB=False, crop=False))
output = net.forward()
H = output.shape[2]
W = output.shape[3]
for i in range(15):
probMap = output[0, i, :, :]
minVal, prob, minLoc, point = cv2.minMaxLoc(probMap)
x = (inWidth * point[0]) / W
y = (inHeight * point[1]) / H
if prob > threshold:
keypoints_list.append((int(x), int(y)))
else:
keypoints_list.append(None)
left_shoulder = keypoints_list[5]
right_shoulder = keypoints_list[2]
top_head = keypoints_list[0]
if left_shoulder is not None and right_shoulder is not None and top_head is not None:
x1 = left_shoulder[0]
y1 = top_head[1]
x2 = right_shoulder[0]
y2 = y1 + (x2 - x1)
cropped_img = upper_body_img[y1:y2, x1:x2]
cv2.imshow('image', cropped_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行代码后,就可以看到剪切后的人像图片。
阅读全文
相关推荐
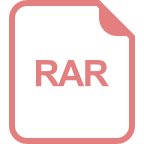
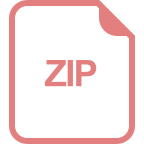
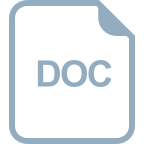
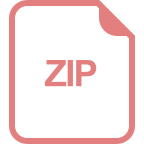
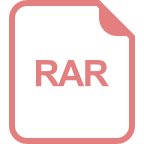
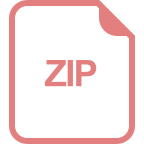
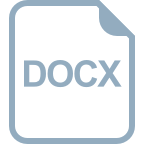
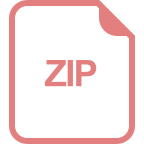
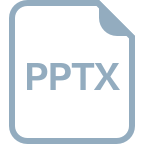
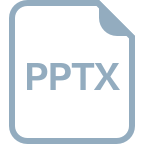
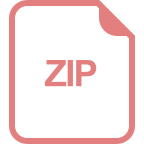
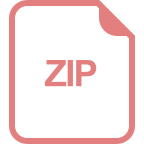
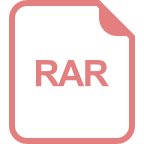
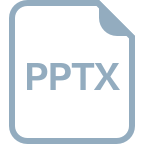