def image_processing(img,device): #img = cv2.resize(img, (32,32)) #img = np.reshape(img, (32, 32, 3)) # normalize img = img.astype(np.float32) img = (img / 255. - mean_value) / std_value img = img.transpose([2, 0, 1]) img = torch.from_numpy(img) img = img.to(device) img = img.view(1, *img.size()) return img
时间: 2023-06-27 22:03:32 浏览: 180
这段代码是一个用于图像处理的函数,输入参数为原始图像和设备类型(CPU或GPU),返回值为经过预处理后的图像张量。
首先,该函数将输入的图像进行缩放和重构,使其变为32x32x3的三维数组,其中32x32是图像的尺寸,3代表RGB颜色通道。接着,函数对图像进行了标准化处理,即将像素值从[0, 255]范围转换到[-1, 1]范围,并且减去均值(mean_value)并除以标准差(std_value)。这是为了使得图像数据更加稳定,方便神经网络进行训练。然后,函数将图像的维度进行转换,使其变为通道维度在前,高度维度在中间,宽度维度在最后的形式。接着,函数将图像数据转换为PyTorch张量,并将其移动到指定的设备上。最后,函数将图像张量的形状变为1x3x32x32的四维数组,并返回该张量。
相关问题
def image_processing(img,device): img = cv2.resize(img, (168,48)) img = np.reshape(img, (48, 168, 3)) # normalize img = img.astype(np.float32) img = (img / 255. - mean_value) / std_value img = img.transpose([2, 0, 1]) img = torch.from_numpy(img) img = img.to(device) img = img.view(1, *img.size()) return img
这段代码是图像处理的函数,它实现的功能是将原始图像进行大小重置、归一化处理和转换成 PyTorch 的张量格式。具体来说,该函数实现了以下几个步骤:
1. 使用 OpenCV 库中的 cv2.resize() 函数将原始图像重置为大小为 (168, 48) 的图像。
2. 将图像的像素值从 [0, 255] 范围归一化到 [-1, 1] 范围,以便于神经网络的处理。这里使用了预先计算好的均值和标准差值进行归一化,mean_value 和 std_value 分别代表均值和标准差。
3. 将图像的维度顺序从 (48, 168, 3) 转换为 (3, 48, 168) 的格式,以符合 PyTorch 的输入格式要求。
4. 将图像转换成 PyTorch 的张量格式,并将其移动到指定的设备上(例如 CPU 或 GPU)。
5. 将图像的维度扩展一维,以符合神经网络的输入要求。
最终,该函数返回处理后的图像张量。
阅读全文
相关推荐
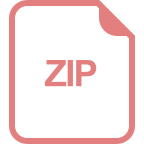
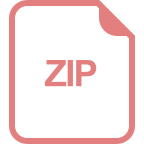
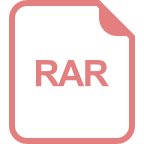
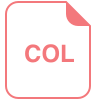
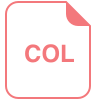
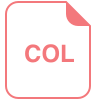
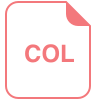
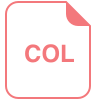
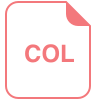
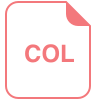
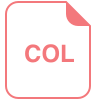
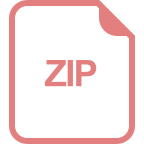
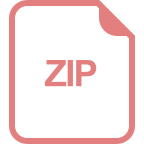