{ "result":true, "code":200, "data":{ "count":6, "success":true, "table":[ { "Availability":100, "Cloud":1, "Col":1182, "DataSourceID":"L1A0003869534", "Date":2019030724, "ID":"1105250", "Level":8, "Provider":"", "Row":518, "Satellite":"GF1", "Sensor":"WFV1", "Size":"", "TileFileName":"GF1_WFV1_2019030724_L1A0003869534_6401_8_518_1182", "Time":"", "type":"Preview", "types":"1,2,3,4" }, { "Availability":100, "Cloud":0, "Col":1182, "DataSourceID":"L1A0006618962", "Date":2022072324, "ID":"1105293", "Level":8, "Provider":"", "Row":518, "Satellite":"GF1", "Sensor":"WFV1", "Size":"", "TileFileName":"GF1_WFV1_2022072324_L1A0006618962_6400_8_518_1182", "Time":"", "type":"Preview", "types":"1,2,3,4" } ] } }使用C#创建一个输出以上json的http接口
时间: 2023-07-03 20:26:48 浏览: 109
可以使用ASP.NET Core来创建一个输出以上JSON的HTTP接口。以下是示例代码:
1. 定义数据模型
```csharp
public class Tile
{
public int Availability { get; set; }
public int Cloud { get; set; }
public int Col { get; set; }
public string DataSourceID { get; set; }
public long Date { get; set; }
public string ID { get; set; }
public int Level { get; set; }
public string Provider { get; set; }
public int Row { get; set; }
public string Satellite { get; set; }
public string Sensor { get; set; }
public string Size { get; set; }
public string TileFileName { get; set; }
public string Time { get; set; }
public string Type { get; set; }
public string Types { get; set; }
}
public class TileResponse
{
public bool Result { get; set; }
public int Code { get; set; }
public TileData Data { get; set; }
}
public class TileData
{
public int Count { get; set; }
public bool Success { get; set; }
public List<Tile> Table { get; set; }
}
```
2. 创建HTTP接口
```csharp
using Microsoft.AspNetCore.Mvc;
using Newtonsoft.Json;
using System.Collections.Generic;
[ApiController]
[Route("[controller]")]
public class TileController : ControllerBase
{
[HttpGet]
public string Get()
{
var tile1 = new Tile
{
Availability = 100,
Cloud = 1,
Col = 1182,
DataSourceID = "L1A0003869534",
Date = 2019030724,
ID = "1105250",
Level = 8,
Provider = "",
Row = 518,
Satellite = "GF1",
Sensor = "WFV1",
Size = "",
TileFileName = "GF1_WFV1_2019030724_L1A0003869534_6401_8_518_1182",
Time = "",
Type = "Preview",
Types = "1,2,3,4"
};
var tile2 = new Tile
{
Availability = 100,
Cloud = 0,
Col = 1182,
DataSourceID = "L1A0006618962",
Date = 2022072324,
ID = "1105293",
Level = 8,
Provider = "",
Row = 518,
Satellite = "GF1",
Sensor = "WFV1",
Size = "",
TileFileName = "GF1_WFV1_2022072324_L1A0006618962_6400_8_518_1182",
Time = "",
Type = "Preview",
Types = "1,2,3,4"
};
var data = new TileData
{
Count = 6,
Success = true,
Table = new List<Tile> { tile1, tile2 }
};
var response = new TileResponse
{
Result = true,
Code = 200,
Data = data
};
return JsonConvert.SerializeObject(response);
}
}
```
3. 运行程序并访问HTTP接口
启动应用程序并访问http://localhost:5000/tile,你将会看到输出了以上JSON数据。
相关推荐
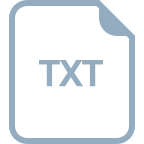
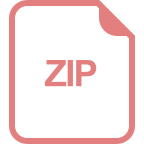
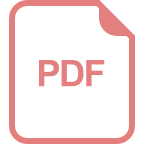
















