设计一个程序,要求利用 read 函数读取系统文件“/etc/passwd”,并在终端 中显示输出。
时间: 2024-05-09 18:20:16 浏览: 16
以下是Python的实现代码:
```python
with open('/etc/passwd', 'r') as f:
content = f.read()
print(content)
```
该代码首先使用 `open` 函数打开 `/etc/passwd` 文件并以只读模式读取文件内容,然后使用 `print` 函数将文件内容输出到终端。
相关问题
用LinuxC语言设计一处程序要求从系统文件“/etc/passwd”读取偏移量从100至200之间的字符,写入“/tmp/pass”文件。
以下是一个简单的C程序,实现了你想要的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#define BUF_SIZE 1024
int main() {
int fd1, fd2;
char buf[BUF_SIZE];
ssize_t nread;
// 打开 /etc/passwd 文件
if ((fd1 = open("/etc/passwd", O_RDONLY)) < 0) {
perror("open /etc/passwd failed");
exit(1);
}
// 创建 /tmp/pass 文件
if ((fd2 = open("/tmp/pass", O_WRONLY | O_CREAT | O_TRUNC, 0644)) < 0) {
perror("create /tmp/pass failed");
exit(1);
}
// 读取文件偏移量在 100 至 200 之间的字符,并写入 /tmp/pass 文件
lseek(fd1, 100, SEEK_SET);
while ((nread = read(fd1, buf, BUF_SIZE)) > 0) {
if (lseek(fd1, 100, SEEK_CUR) == -1) {
perror("lseek failed");
exit(1);
}
if (write(fd2, buf, nread) != nread) {
perror("write failed");
exit(1);
}
if (lseek(fd1, 100, SEEK_CUR) == -1) {
perror("lseek failed");
exit(1);
}
}
if (nread == -1) {
perror("read failed");
exit(1);
}
// 关闭文件
close(fd1);
close(fd2);
return 0;
}
```
这个程序首先打开了 /etc/passwd 文件和 /tmp/pass 文件,然后使用 lseek 函数将文件指针移到偏移量为 100 的位置开始读取,并将读到的字符写入 /tmp/pass 文件中。最后关闭了两个文件。注意,这里使用了一些错误处理来确保程序的正确性。
生成代码设计一个程序,要求打开文件“pass”,如果没有这个文件,新建此文件;读取系统文件“etc/passwd”,把文件内容复制到“pass”文件中。
以下是 Python 代码实现:
```python
import os
# 检查 "pass" 文件是否存在,如果不存在则新建
if not os.path.exists("pass"):
open("pass", "w").close()
# 读取系统文件 "etc/passwd"
with open("/etc/passwd", "r") as f:
content = f.read()
# 把文件内容复制到 "pass" 文件中
with open("pass", "w") as f:
f.write(content)
```
代码逻辑如下:
1. 使用 `os.path.exists` 函数检查当前目录下是否存在 "pass" 文件。
2. 如果 "pass" 文件不存在,则使用 `open` 函数新建该文件。
3. 使用 `with open` 语句打开系统文件 "/etc/passwd" 并读取其内容。
4. 使用 `with open` 语句打开 "pass" 文件并将读取的内容写入该文件中。
相关推荐
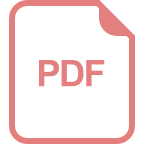
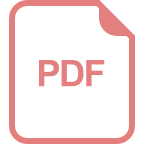
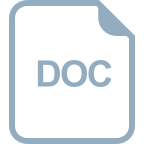









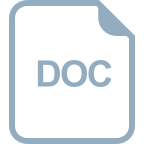
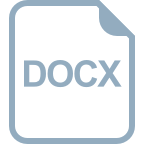
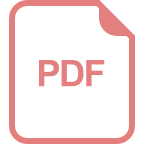
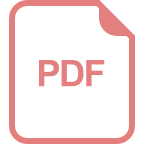