java编写一个方法功能是求小数或整数的绝对值
时间: 2024-02-15 07:01:33 浏览: 26
同样可以使用Math类中的abs()方法来求小数或整数的绝对值。下面是一个示例代码:
```java
public class Main {
public static void main(String[] args) {
int num1 = -10;
double num2 = -3.14;
int absNum1 = Math.abs(num1);
double absNum2 = Math.abs(num2);
System.out.println("The absolute value of " + num1 + " is " + absNum1);
System.out.println("The absolute value of " + num2 + " is " + absNum2);
}
}
```
输出结果为:
```
The absolute value of -10 is 10
The absolute value of -3.14 is 3.14
```
相关问题
编写一个方法abs( ),既可以对整数进行绝对值获取,又可以对小数进行绝对值的获取
可以使用Python内置函数abs()来获取整数或小数的绝对值。例如:
```python
def abs(num):
return abs(num)
```
这样编写的abs()方法可以对整数或小数进行绝对值的获取。如果传入的是整数,那么返回的就是这个整数的绝对值;如果传入的是小数,那么返回的就是这个小数的绝对值。
编写一个Java类分别实现求三个整数中的最大数和最小数
```java
public class MaxMinFinder {
public static int findMax(int a, int b, int c) {
int max = a;
if (b > max) {
max = b;
}
if (c > max) {
max = c;
}
return max;
}
public static int findMin(int a, int b, int c) {
int min = a;
if (b < min) {
min = b;
}
if (c < min) {
min = c;
}
return min;
}
}
```
使用示例:
```java
int a = 10, b = 20, c = 30;
int max = MaxMinFinder.findMax(a, b, c);
int min = MaxMinFinder.findMin(a, b, c);
System.out.println("Max: " + max); // 输出 "Max: 30"
System.out.println("Min: " + min); // 输出 "Min: 10"
```
相关推荐
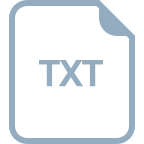
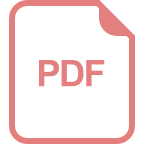












