前端接受字符串时间转localdateTime时间注解
时间: 2023-08-13 10:05:55 浏览: 49
在JavaScript中,可以使用Date对象来处理日期和时间。如果你要将一个字符串转换为LocalDateTime时间,你可以使用以下代码:
```javascript
// 假设你有一个字符串时间 "2021-06-30T12:30:00"
const dateString = "2021-06-30T12:30:00";
// 使用Date对象将字符串时间转换为LocalDateTime时间
const localDateTime = new Date(dateString);
// 打印结果
console.log(localDateTime);
```
这将输出一个LocalDateTime对象,例如:
```
Wed Jun 30 2021 12:30:00 GMT+0800 (中国标准时间)
```
如果你想要以不同的格式显示LocalDateTime时间,可以使用Date对象的一些方法,例如getDate()、getMonth()、getFullYear()、getHours()、getMinutes()、getSeconds()等。
相关问题
springboo将前端字符串转化成localdatetime
在SpringBoot中将前端字符串转化成LocalDateTime,我们可以使用@DateTimeFormat注解和LocalDateTime.parse()方法。
首先,我们需要在实体类的相应属性上添加@DateTimeFormat注解,以指示SpringBoot将在绑定时使用的日期格式。例如:
```java
import org.springframework.format.annotation.DateTimeFormat;
public class MyEntity {
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss")
private LocalDateTime myDate;
// getters and setters
}
```
上面的示例中,我们指示SpringBoot使用以“yyyy-MM-dd HH:mm:ss”格式的日期字符串来绑定myDate属性。
然后,我们可以在我们的控制器中使用以下代码将前端传递的日期字符串转换为LocalDateTime:
```java
import java.time.LocalDateTime;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
public class MyController {
// request body example: {"myDate": "2022-10-09 20:22:33"}
@PostMapping("/my-endpoint")
public void myEndpoint(@RequestBody MyEntity myEntity) {
LocalDateTime myDate = LocalDateTime.parse(myEntity.getMyDate());
// do something with myDate
}
}
```
在上面的示例中,我们使用LocalDateTime.parse()方法将从前端收到的日期字符串myEntity.getMyDate()转换为LocalDateTime格式。
最后,我们可以在我们的服务类中使用LocalDateTime格式的日期执行业务逻辑。
LocalDateTime属性值前端显示带T 加了LocalDateTime也没用
根据提供的两个引用,可以看出在Java中使用LocalDateTime类型时,需要注意两个问题:1.在LocalDateTime字段上添加@JsonSerialize(using = LocalDateTimeConverter.class)注解;2.在LocalDateTime字段上添加@JsonFormat(shape=JsonFormat.Shape.STRING, pattern="yyyy-MM-dd HH:mm:ss")注解。其中,第一个注解是为了将LocalDateTime类型转换为JSON字符串,第二个注解是为了将LocalDateTime类型以指定格式化日期的方式返回给前端。如果只添加第一个注解,前端会默认将LocalDateTime类型解析为带T的字符串,而不是指定的日期格式。因此,需要同时添加这两个注解才能正确地将LocalDateTime类型转换为指定格式的日期字符串。
以下是一个示例代码,演示如何在Java中使用LocalDateTime类型并正确地将其转换为指定格式的日期字符串:
```java
import java.time.LocalDateTime;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.datatype.jsr310.ser.LocalDateTimeSerializer;
public class Example {
@JsonSerialize(using = LocalDateTimeSerializer.class)
@JsonFormat(shape=JsonFormat.Shape.STRING, pattern="yyyy-MM-dd HH:mm:ss")
protected LocalDateTime createTime;
public Example(LocalDateTime createTime) {
this.createTime = createTime;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
}
// 在Controller中使用示例
@RestController
public class ExampleController {
@GetMapping("/example")
public Example getExample() {
LocalDateTime createTime = LocalDateTime.now();
return new Example(createTime);
}
}
```
相关推荐
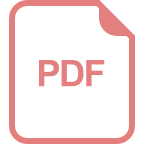











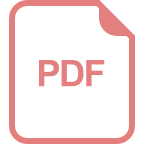