如何在WinForm from1中的splitContainer1.Panel1控件上制作一个包含父级菜单名称和子级功能选项的功能选项栏(父级菜单可点击展开显示所有子级,一个父级菜单包含多个子级功能), 并在splitContainer1.Panel1顶部增加一个功能搜索栏(根据输入的子级功能名称高亮显示出来对应的功能),点击对应的子级功能调用From2窗体填充到stabControl1里的tabControl界面(每打开一个子级功能自动新增一个TabPages界面TabPages文本显示点击的子级功能名称,TabPages名称右边包含关闭(X)功能,点击之后关闭调用的from2窗体)同样的再点击其他子级功能调用其他的from3窗体,以此类推 子级功能要和From对应起来。实现代码
时间: 2024-03-14 12:48:01 浏览: 20
好的,以下是一个实现这个功能的代码。
首先,我们需要在splitContainer1.Panel1中添加一个TreeView控件,用于展示父级菜单和子级功能选项。同时,在splitContainer1.Panel1的顶部添加一个TextBox控件,用于输入子级功能名称进行搜索。
```
private TreeView treeView1;
private TextBox textBox1;
public Form1()
{
InitializeComponent();
// 初始化TreeView控件
treeView1 = new TreeView();
treeView1.Dock = DockStyle.Fill;
treeView1.AfterSelect += TreeView1_AfterSelect;
splitContainer1.Panel1.Controls.Add(treeView1);
// 初始化TextBox控件
textBox1 = new TextBox();
textBox1.Dock = DockStyle.Top;
textBox1.TextChanged += TextBox1_TextChanged;
splitContainer1.Panel1.Controls.Add(textBox1);
}
```
接下来,我们需要在TreeView中添加父级菜单和子级功能选项。这里我们可以先通过一个二维数组来模拟数据,可以根据实际情况进行修改。
```
private string[,] menuItems = {
{ "菜单1", "功能1", "功能2", "功能3" },
{ "菜单2", "功能4", "功能5", "功能6" },
{ "菜单3", "功能7", "功能8", "功能9" }
};
private void Form1_Load(object sender, EventArgs e)
{
// 添加父级菜单节点
for (int i = 0; i < menuItems.GetLength(0); i++)
{
TreeNode parentNode = new TreeNode(menuItems[i, 0]);
treeView1.Nodes.Add(parentNode);
// 添加子级功能节点
for (int j = 1; j < menuItems.GetLength(1); j++)
{
TreeNode childNode = new TreeNode(menuItems[i, j]);
parentNode.Nodes.Add(childNode);
}
}
}
```
然后,我们需要根据子级功能名称来打开对应的From窗体。我们可以使用一个字典来存储子级功能名称和对应的From窗体类型。在TreeView的节点点击事件中,根据选中节点的文本来从字典中获取对应的From窗体类型,并创建一个新的From窗体实例,并将其添加到stabControl1中。
```
private Dictionary<string, Type> formTypes = new Dictionary<string, Type>
{
{ "功能1", typeof(Form2) },
{ "功能2", typeof(Form3) },
{ "功能3", typeof(Form4) },
{ "功能4", typeof(Form5) },
{ "功能5", typeof(Form6) },
{ "功能6", typeof(Form7) },
{ "功能7", typeof(Form8) },
{ "功能8", typeof(Form9) },
{ "功能9", typeof(Form10) },
};
private void TreeView1_AfterSelect(object sender, TreeViewEventArgs e)
{
if (e.Node.Level == 1)
{
// 获取选中的子级功能名称
string childNodeText = e.Node.Text;
// 根据子级功能名称从字典中获取对应的From窗体类型
if (formTypes.TryGetValue(childNodeText, out Type formType))
{
// 创建新的From窗体实例并添加到stabControl1中
Form form = (Form)Activator.CreateInstance(formType);
form.TopLevel = false;
form.FormBorderStyle = FormBorderStyle.None;
form.Dock = DockStyle.Fill;
TabPage tabPage = new TabPage(childNodeText);
tabPage.Tag = form;
tabPage.Controls.Add(form);
tabPage.Controls.Add(new Button()
{
Text = "X",
Dock = DockStyle.Right,
Width = 30,
Tag = tabPage,
});
tabPage.Controls[1].Click += TabPage_CloseButtonClick;
stabControl1.TabPages.Add(tabPage);
stabControl1.SelectedTab = tabPage;
form.Show();
}
}
}
```
最后,我们需要实现TabPages的关闭功能。我们在每个TabPage中添加一个Button控件,用于关闭TabPage。在Button的Click事件中,我们将对应的From窗体关闭,并从stabControl1中移除该TabPage。
```
private void TabPage_CloseButtonClick(object sender, EventArgs e)
{
Button closeButton = (Button)sender;
TabPage tabPage = (TabPage)closeButton.Tag;
Form form = (Form)tabPage.Tag;
form.Close();
stabControl1.TabPages.Remove(tabPage);
}
```
以上就是一个完整的实现代码,你可以根据自己的需求进行修改和优化。
相关推荐
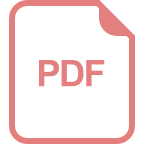














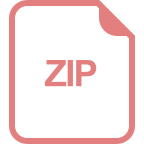
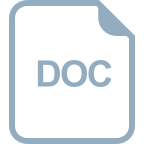