Force Unwrapping Violation: Force unwrapping should be avoided. (force_unwrapping)
时间: 2024-03-11 13:50:36 浏览: 21
Force unwrapping is a term used in programming to refer to the act of using the exclamation mark (!) to force the unwrapping of an optional value. For example, if we have an optional variable called "name" and we want to use its value, we can force unwrap it like this:
```
let name: String? = "John Doe"
let unwrappedName = name!
```
However, using force unwrapping can be dangerous because if the optional value is nil, it will cause a runtime error and crash the program. Therefore, it is generally recommended to avoid force unwrapping and instead use optional binding or optional chaining to safely handle optional values.
相关问题
Force Unwrapping Violation: Force unwrapping should be avoided. (force_unwrapping) 如何解决
To resolve the force unwrapping violation, you can do the following:
1. Replace the force unwrapping with optional binding:
Instead of using the exclamation mark to force unwrap an optional value, you can use optional binding to safely unwrap the value. For example:
```
let name: String? = "John Doe"
if let unwrappedName = name {
// Use unwrappedName
} else {
// Handle nil case
}
```
2. Use optional chaining:
Optional chaining is another way to safely handle optional values without force unwrapping. For example:
```
let name: String? = "John Doe"
let unwrappedName = name?.uppercased()
```
In this example, if the name is nil, the result of the expression will also be nil, without causing a runtime error.
3. Use guard statements:
Guard statements can also be used to safely unwrap optional values and handle the nil case. For example:
```
func doSomething(withName name: String?) {
guard let unwrappedName = name else {
// Handle nil case
return
}
// Use unwrappedName
}
```
In this example, if the name is nil, the guard statement will exit the function and handle the nil case. If the name is not nil, the unwrappedName constant will be available for use within the function.
a=load('re_phase_unwrapping.mat'); phase_unwrapping=a.re_phase_unwrapping; 这段代码中a.re_phase_unwrapping是什么意思
这段代码中,a是一个MATLAB文件的加载结果,其中包含变量re_phase_unwrapping。re_phase_unwrapping是MATLAB文件中保存的一个变量,它可能是一个数值、向量、矩阵或其他类型的数据。此处的代码将变量re_phase_unwrapping从MATLAB文件中加载到当前的工作空间中,以便在后续的代码中使用。
相关推荐
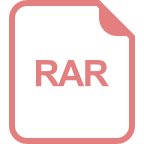












