写一个应用pthread_create和pthread_detach的代码
时间: 2023-09-11 18:09:56 浏览: 49
当然可以!这是一个示例代码,演示了如何使用`pthread_create()`和`pthread_detach()`函数创建和分离线程。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_func(void *arg) {
int thread_num = *(int*)arg;
printf("Hello from thread %d\n", thread_num);
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int thread_num = 1;
// 创建线程
if (pthread_create(&thread, NULL, thread_func, &thread_num) != 0) {
fprintf(stderr, "Failed to create thread\n");
return 1;
}
// 分离线程
if (pthread_detach(thread) != 0) {
fprintf(stderr, "Failed to detach thread\n");
return 1;
}
// 继续主线程的工作
printf("Hello from main thread\n");
// 等待一段时间确保子线程完成
sleep(1);
return 0;
}
```
在上面的示例中,我们使用`pthread_create()`函数创建了一个新的线程,并将其绑定到`thread`变量中。该线程执行`thread_func()`函数,它接受一个指向`thread_num`的指针作为参数,并在屏幕上打印相关信息。
接下来,我们使用`pthread_detach()`函数将线程与主线程分离。这意味着主线程不会等待子线程的终止,而是允许它自行运行并释放资源。
最后,主线程打印自己的信息,并通过调用`sleep()`函数等待一段时间,以确保子线程有足够的时间完成执行。然后,程序返回0,表示成功运行。
请注意,在实际编写使用多线程的应用程序时,可能需要更复杂的同步和线程管理机制来确保正确的执行顺序和资源管理。以上代码仅提供了一个简单的示例来演示`pthread_create()`和`pthread_detach()`的用法。
相关推荐
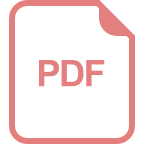
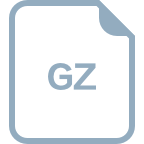
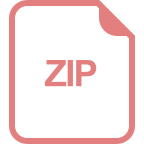














