qtc++实现一个用户账户类,类中包括保存用户的账号名和密码,包含设置账号和密码、获取账号和密码的成员函数,编写主函数测试类的使用。
时间: 2024-12-22 16:13:03 浏览: 7
在C++中,我们可以创建一个名为`UserAccount`的基本类来表示用户账户,包含私有变量存储用户名和密码,以及公共方法来操作这些信息。这是一个简单的例子:
```cpp
#include <iostream>
#include <string>
class UserAccount {
private:
std::string username;
std::string password;
public:
// 构造函数
UserAccount(const std::string& username, const std::string& password) :
username(username), password(password) {}
// 设置账号和密码
void setCredentials(const std::string& newUsername, const std::string& newPassword) {
username = newUsername;
password = newPassword;
}
// 获取账号
std::string getUsername() const {
return username;
}
// 获取密码 (注意这里通常会将密码视为敏感信息,在实际应用中不应直接返回)
std::string getPassword() const {
return "****"; // 返回星号或其他占位符代替真实密码
}
};
int main() {
// 创建一个用户账户
UserAccount user("JohnDoe", "mypassword");
// 测试设置和获取功能
std::cout << "Original Username: " << user.getUsername() << "\n";
user.setCredentials("JaneDoe", "newpassword");
std::cout << "Updated Username: " << user.getUsername() << "\n";
// 获取密码,仅展示处理敏感信息的方式
std::cout << "Password: " << user.getPassword() << "\n"; // 输出星号代替
return 0;
}
```
在这个例子中,我们创建了一个`UserAccount`类,它有两个私有成员变量`username`和`password`,并提供`setCredentials`和`getUsername`、`getPassword`方法用于设置和获取数据。在`main`函数中,我们实例化了这个类,并演示了如何使用这些方法。
阅读全文
相关推荐
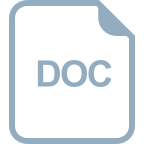
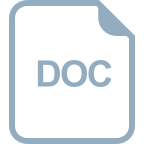
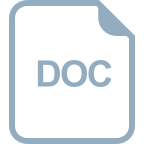
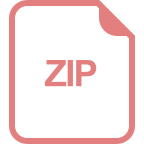

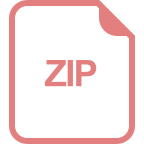
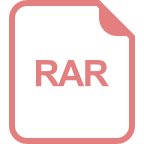
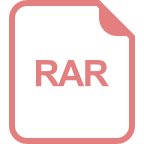
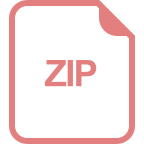
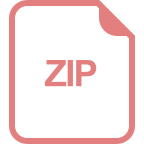
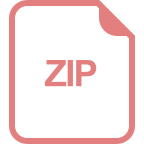
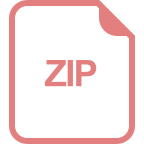
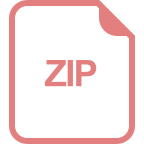
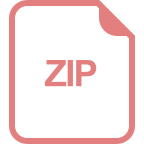
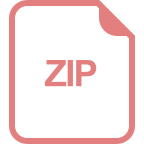
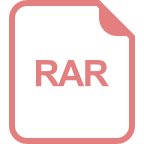
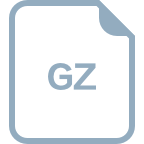
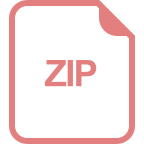
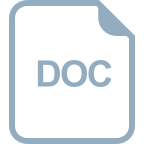