将字母转换成大写的函数
时间: 2024-10-23 20:14:27 浏览: 23
将字母转换成大写通常是在编程中处理文本操作时常见的需求,很多编程语言都内置了这样的功能。例如,在Python中,你可以使用`upper()`函数,如下所示:
```python
def to_upper_case(text):
return text.upper()
# 示例
input_string = "hello world"
output_string = to_upper_case(input_string)
print(output_string) # 输出: HELLO WORLD
```
在JavaScript中,也有相似的`toUpperCase()`方法:
```javascript
function toUpperCase(str) {
return str.toUpperCase();
}
// 示例
let inputString = "hello world";
let outputString = toUpperCase(inputString);
console.log(outputString); // 输出: HELLO WORLD
```
其他语言如C#、Java等也都有相应的字符串操作函数可以实现字符转大写。
相关问题
python中将小写字母转换成大写字母的函数
在Python中,可以使用字符串对象的`upper()`方法将小写字母转换为大写字母。例如:
```
string = "hello world"
upper_string = string.upper()
print(upper_string)
```
输出结果为:
```
HELLO WORLD
```
另外,还可以使用内置函数`str.upper()`将字符转换成大写字母,例如:
```
char = 'a'
upper_char = str.upper(char)
print(upper_char)
```
输出结果为:
```
A
```
不使用函数将小写字母转换成大写字母
可以使用ASCII码来将小写字母转换成大写字母。ASCII码中,字符'A'到'Z'的值依次为65到90,字符'a'到'z'的值依次为97到122。因此,将小写字母转换成大写字母的方法是将其ASCII码值减去32。以下是一个简单的示例代码:
```
#include <iostream>
using namespace std;
int main() {
char ch;
cout << "请输入一个小写字母:";
cin >> ch;
if (ch >= 'a' && ch <= 'z') {
ch -= 32;
}
cout << "转换后的大写字母为:" << ch << endl;
return 0;
}
```
注意,此方法只适用于ASCII码编码的字符集,不适用于其他编码方式的字符集。
阅读全文
相关推荐
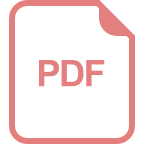
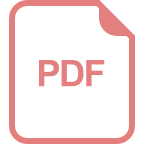
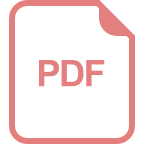













