asp .net core怎么使用Redis缓存获取个人信息
时间: 2024-10-05 22:01:12 浏览: 39
在ASP.NET Core中使用Redis作为缓存存储个人信息,通常涉及以下步骤:
1. **安装依赖**:首先需要添加Microsoft.Extensions.Caching.Redis NuGet包到项目中,以使用Redis缓存功能。
```shell
dotnet add package Microsoft.Extensions.Caching.Redis
```
2. **配置连接**:在`appsettings.json`文件中添加Redis服务器的连接字符串,并在`Startup.cs`中的ConfigureServices方法里注入`IOptions<RedisCacheOptions>`,以便在运行时获取配置。
```json
{
"Redis": {
"ConnectionString": "your_redis_connection_string_here"
}
}
```
```csharp
services.AddStackExchangeRedis(options =>
{
options.Configuration = Configuration.GetSection("Redis");
});
```
3. **创建服务**:定义一个RedisCacheService,它将使用注入的`IRedisCache`来操作缓存。
```csharp
public class RedisCacheService
{
private readonly IRedisCache _redisCache;
public RedisCacheService(IRedisCache redisCache)
{
_redisCache = redisCache;
}
// 示例方法获取用户信息
public async Task<User> GetUserFromCache(string userId)
{
var userInfo = await _redisCache.GetAsync(userId);
if (userInfo != null) return JsonConvert.DeserializeObject<User>(userInfo.ToString());
else // 如果未找到缓存,返回默认值或从数据库查询
return CreateUserIfNotFound(userId);
}
private User CreateUserIfNotFound(string userId)
{
// 实现根据userId查询数据库的操作
// 返回User对象
}
}
```
4. **使用服务**:在控制器或业务层中注入`RedisCacheService`,并利用其方法获取用户信息。
```csharp
public class UserController
{
private readonly RedisCacheService _cacheService;
public UserController(RedisCacheService cacheService)
{
_cacheService = cacheService;
}
[HttpGet("{userId}")]
public async Task<IActionResult> GetUser(string userId)
{
User user = await _cacheService.GetUserFromCache(userId);
// 如果缓存中无数据,处理未命中情况,如返回500等
return Ok(user);
}
}
```
阅读全文
相关推荐
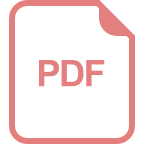
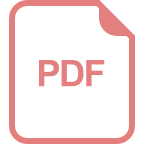
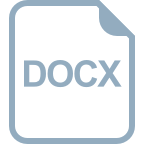
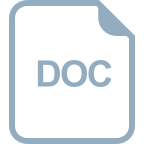
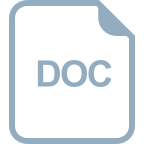
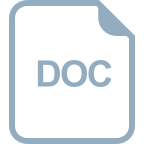
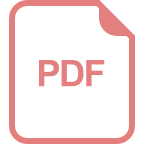
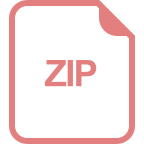
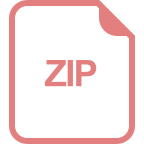
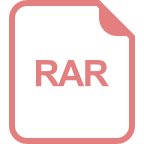
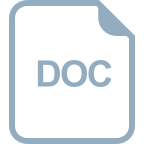
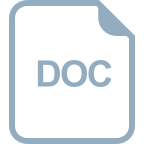
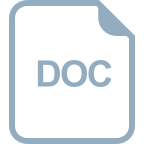
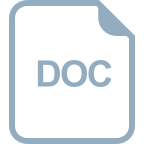
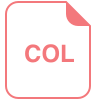
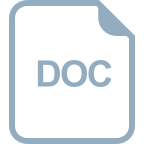