python医学三维图
时间: 2023-08-25 12:10:31 浏览: 43
在Python中,您可以使用一些库来创建医学三维图。以下是一些常用的库和示例代码:
1. Matplotlib:Matplotlib是一个广泛使用的绘图库,可以用于创建各种类型的图表,包括三维图。以下是一个简单的例子:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 创建数据
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
# 创建图形和坐标轴
fig = plt.figure()
ax = fig.add_subplot(111
相关问题
python开发三维dicom
Python开发三维DICOM(数字成像与通信医学)的过程是将DICOM图像数据加载到Python环境中,并使用适当的工具和库来处理和呈现这些数据。
在Python中,可以使用pydicom库来读取和解析DICOM文件。通过调用pydicom库的相关函数,可以轻松地加载DICOM图像数据,并访问图像的元数据和像素值。
一旦DICOM数据被加载到Python环境中,就可以使用其他库,如NumPy和matplotlib,来处理和可视化三维DICOM图像。NumPy库提供了强大的数组操作功能,可以用于对DICOM图像进行各种数学和几何运算。而matplotlib库可以用于创建各种类型的图形,包括三维图像和交互式的视图。
为了生成三维DICOM图像,可以使用现成的可视化工具,如Mayavi和VTK(可视化工具包)。Mayavi是一个使用VTK库的高级数据可视化工具,可以轻松地创建三维DICOM图像并进行交互式演示。
在Python开发三维DICOM过程中,需要注意以下几点:
1. 熟悉DICOM格式和结构,以便正确地读取和解析DICOM文件。
2. 了解NumPy和matplotlib库的使用方法,以便进行图像处理和可视化。
3. 了解Mayavi或VTK库的使用方法,以便创建三维DICOM图像和交互式视图。
总结起来,Python开发三维DICOM需要使用pydicom库来加载DICOM数据,NumPy和matplotlib库进行图像处理和可视化,Mayavi或VTK库创建三维图像。熟悉这些工具和库的使用方法,并了解DICOM数据的特点和结构,可以帮助顺利完成三维DICOM开发任务。
python 器官三维重建
Python 是一种广泛应用于科学计算和数据处理的编程语言。在医学领域,Python 可以用于器官三维重建。器官三维重建是指通过处理医学影像数据,如 CT、MRI 或超声波图像,生成器官的三维模型。
Python 提供了一系列强大的库和工具,用于医学影像数据的处理和分析。其中最常用的库是 NumPy 和 SciPy,它们提供了高效的矩阵运算和科学计算功能。另外,还有 Pandas 用于数据处理,Matplotlib 和 seaborn 用于数据可视化,Scikit-learn 用于机器学习等。
在器官三维重建中,首先需要导入医学影像数据。这可以通过 PyDICOM 库来读取 DICOM 格式的图像数据。然后,可以通过 NumPy 将图像数据转换为三维数组,方便进行处理。
接下来,可以使用一些图像处理算法来提取感兴趣的器官。比如,可以使用阈值分割算法将器官与周围组织分割开来。还可以使用形态学操作来去除噪声和平滑图像。
在进行器官三维重建时,可以使用三维可视化库,如 VTK 或 Mayavi,来将二维图像堆叠成三维模型。这些库提供了各种可视化功能,如体渲染、表面重建和体绘制等。
最后,可以使用这些三维模型进行进一步的分析和研究。比如,可以通过计算体积,评估器官的大小和形状变化。也可以利用模型进行手术模拟、病灶定位和疾病诊断等。
总之,Python 提供了丰富的工具和库,使得器官三维重建变得更加简单和高效。它在医学领域的应用潜力巨大,可以帮助医生进行更精确的诊断和治疗。
相关推荐
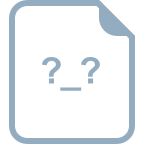
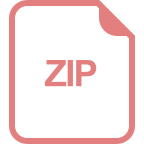
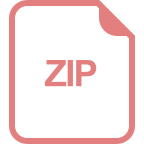












