vue element ui 写一个富文本编辑器 加粗标红
时间: 2023-12-09 18:35:43 浏览: 78
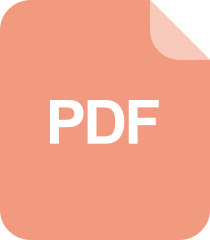
Vue+Element UI+vue-quill-editor富文本编辑器及插入图片自定义
可以使用Vue和Element UI来实现一个富文本编辑器,同时也可以使用第三方库Quill来实现。以下是使用Element UI和Quill实现的代码示例:
使用Element UI:
1. 首先需要安装Element UI和Quill:
```
npm install element-ui quill --save
```
2. 在Vue组件中引入Element UI和Quill:
```javascript
import { quillEditor } from 'vue-quill-editor'
import 'quill/dist/quill.core.css'
import 'quill/dist/quill.snow.css'
import 'quill/dist/quill.bubble.css'
import { Button, Input } from 'element-ui'
import 'element-ui/lib/theme-chalk/index.css'
```
3. 在Vue组件中使用Element UI和Quill:
```html
<template>
<div>
<el-button type="primary" @click="bold">加粗</el-button>
<el-button type="primary" @click="red">标红</el-button>
<quill-editor v-model="content" :options="editorOption"></quill-editor>
</div>
</template>
<script>
export default {
components: {
quillEditor,
'el-button': Button,
'el-input': Input
},
data() {
return {
content: '',
editorOption: {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'],
['blockquote', 'code-block'],
[{ 'header': 1 }, { 'header': 2 }],
[{ 'list': 'ordered' }, { 'list': 'bullet' }],
[{ 'script': 'sub' }, { 'script': 'super' }],
[{ 'indent': '-1' }, { 'indent': '+1' }],
[{ 'direction': 'rtl' }],
[{ 'size': ['small', false, 'large', 'huge'] }],
[{ 'header': [1, 2, 3, 4, 5, 6, false] }],
[{ 'color': [] }, { 'background': [] }],
[{ 'font': [] }],
[{ 'align': [] }],
['clean'],
['link', 'image', 'video']
]
}
}
}
},
methods: {
bold() {
const quill = this.$refs.myQuillEditor.quill
const range = quill.getSelection()
if (range) {
quill.format('bold', true)
}
},
red() {
const quill = this.$refs.myQuillEditor.quill
const range = quill.getSelection()
if (range) {
quill.format('color', 'red')
}
}
}
}
</script>
```
使用Quill:
1. 首先需要安装Quill:
```
npm install quill --save
```
2. 在Vue组件中引入Quill:
```javascript
import Quill from 'quill'
import 'quill/dist/quill.core.css'
import 'quill/dist/quill.snow.css'
import 'quill/dist/quill.bubble.css'
```
3. 在Vue组件中使用Quill:
```html
<template>
<div>
<button @click="bold">加粗</button>
<button @click="red">标红</button>
<div ref="editor"></div>
</div>
</template>
<script>
export default {
data() {
return {
editor: null
}
},
mounted() {
this.editor = new Quill(this.$refs.editor, {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'],
['blockquote', 'code-block'],
[{ 'header': 1 }, { 'header': 2 }],
[{ 'list': 'ordered' }, { 'list': 'bullet' }],
[{ 'script': 'sub' }, { 'script': 'super' }],
[{ 'indent': '-1' }, { 'indent': '+1' }],
[{ 'direction': 'rtl' }],
[{ 'size': ['small', false, 'large', 'huge'] }],
[{ 'header': [1, 2, 3, 4, 5, 6, false] }],
[{ 'color': [] }, { 'background': [] }],
[{ 'font': [] }],
[{ 'align': [] }],
['clean'],
['link', 'image', 'video']
]
},
theme: 'snow'
})
},
methods: {
bold() {
const range = this.editor.getSelection()
if (range) {
this.editor.format('bold', true)
}
},
red() {
const range = this.editor.getSelection()
if (range) {
this.editor.format('color', 'red')
}
}
}
}
</script>
```
阅读全文
相关推荐
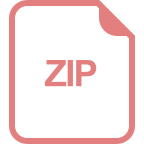
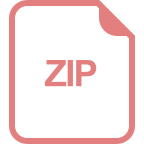









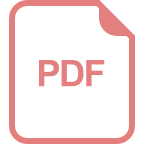
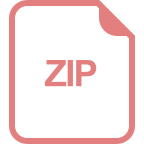
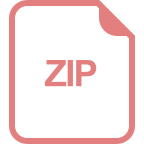
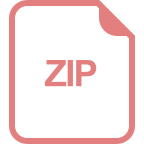
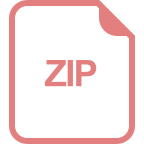
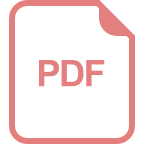