requests库构造请求
时间: 2023-08-12 14:09:38 浏览: 43
requests库是一个常用的HTTP请求库,可以用来发送HTTP请求并获取响应。要构造一个请求,可以使用requests库中的`request`函数或者直接使用对应的HTTP方法函数(如`get`、`post`等)。
下面是使用`request`函数构造一个GET请求的示例:
```python
import requests
url = 'http://www.example.com'
headers = {'User-Agent': 'Mozilla/5.0'}
params = {'param1': 'value1', 'param2': 'value2'}
response = requests.request('GET', url, headers=headers, params=params)
print(response.status_code) # 打印响应状态码
print(response.text) # 打印响应内容
```
在上面的示例中,我们首先定义了请求的URL、请求头和查询参数。然后使用`requests.request`函数构造一个GET请求,并将响应保存在`response`变量中。最后,我们可以通过`response`对象获取响应的状态码和内容。
除了GET请求,requests库还支持其他常用的HTTP方法,如POST、PUT、DELETE等。你可以根据不同的需求选择合适的方法进行请求构造。
相关问题
python requests 构造请求头
在 Python 中使用 requests 库构造请求头可以通过传递一个字典参数给 requests.get() 或 requests.post() 方法的 headers 参数来实现。下面是一个示例代码:
```python
import requests
url = 'http://www.example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36 Edge/16.16299',
'Accept-Language': 'en-US,en;q=0.5',
'Accept-Encoding': 'gzip, deflate',
'Connection': 'keep-alive',
'Referer': 'http://www.example.com/index.html'
}
response = requests.get(url, headers=headers)
print(response.content)
```
在这个示例中,我们定义了一个名为 headers 的字典,其中包含了多个常见的请求头信息。然后,我们将这个字典作为参数传递给了 requests.get() 方法的 headers 参数。最后,我们打印了响应内容。
当然,具体的请求头信息需要根据具体的需求进行调整。
requests库 get请求模拟登录
requests库是一个常用的Python第三方库,用于发送HTTP请求。通过使用requests库,可以方便地进行GET请求模拟登录。
GET请求是一种向服务器获取数据的方式,而模拟登录则是通过发送带有登录信息的请求来模拟用户登录的过程。下面是使用requests库进行GET请求模拟登录的基本步骤:
1. 导入requests库:
```python
import requests
```
2. 构造GET请求的URL和参数:
```python
url = "登录页面的URL"
params = {
"username": "用户名",
"password": "密码"
}
```
3. 发送GET请求:
```python
response = requests.get(url, params=params)
```
4. 处理响应:
```python
if response.status_code == 200:
# 登录成功,可以继续进行其他操作
# 可以通过response.text或response.json()获取响应内容
else:
# 登录失败,可以根据具体情况进行处理
```
需要注意的是,具体的登录过程可能会有一些特殊的要求,比如需要使用POST请求、需要添加请求头等。在实际使用中,可以根据具体的登录页面和要求进行相应的调整。
相关推荐
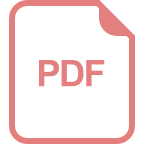
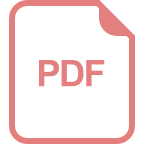
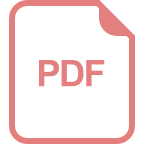












