C语言用for循环求存款
时间: 2024-12-24 08:30:58 浏览: 4
在C语言中,你可以使用for循环来模拟存款操作。假设我们要创建一个简单的程序,让用户输入存款金额并累计总存款,可以按照以下步骤编写:
```c
#include <stdio.h> // 引入输入输出库
int main() {
int deposit; // 存款金额
float total_deposit = 0.0; // 总存款初始值为0
printf("请输入存款金额(输入-1结束):\n");
for (;;) { // 使用无限循环直到用户输入-1停止
scanf("%d", &deposit); // 读取用户的输入
if (deposit == -1) break; // 如果输入-1,跳出循环
total_deposit += deposit; // 累加存款
printf("当前存款总额: %.2f\n", total_deposit); // 输出累计总额
}
return 0;
}
```
在这个例子中,`for`循环会一直运行,直到用户输入 `-1` 表示结束存款。每次迭代都会读取用户的存款金额,并将其加到 `total_deposit` 上。
相关问题
用c语言实现银行存款程序
以下是一个简单的银行存款程序的C语言代码:
```c
#include <stdio.h>
int main() {
double balance = 0.0, amount;
int option;
printf("Welcome to the bank!\n");
while (1) {
printf("\n1. Check balance\n");
printf("2. Deposit\n");
printf("3. Withdraw\n");
printf("4. Quit\n");
printf("Enter your choice: ");
scanf("%d", &option);
switch (option) {
case 1:
printf("Your balance is: %.2f\n", balance);
break;
case 2:
printf("Enter deposit amount: ");
scanf("%lf", &amount);
balance += amount;
printf("Deposit successful! Your new balance is: %.2f\n", balance);
break;
case 3:
printf("Enter withdraw amount: ");
scanf("%lf", &amount);
if (amount > balance) {
printf("Withdrawal unsuccessful! Insufficient balance.\n");
} else {
balance -= amount;
printf("Withdrawal successful! Your new balance is: %.2f\n", balance);
}
break;
case 4:
printf("Thank you for using our bank!\n");
return 0;
default:
printf("Invalid option selected!\n");
break;
}
}
return 0;
}
```
在此程序中,我们使用了一个循环来持续提供银行服务,直到用户选择退出。我们使用了一个 switch 语句来处理用户的选择,包括检查余额、存款、取款和退出。我们还使用了一个变量 balance 来跟踪用户的余额,并且通过简单的算术运算来更新余额。
C语言计算存款的本金合计
以下是一个计算存款本金合计的C语言程序:
```c
#include <stdio.h>
int main() {
float principal, interest;
int years;
printf("Enter the principal amount: ");
scanf("%f", &principal);
printf("Enter the interest rate: ");
scanf("%f", &interest);
printf("Enter the number of years: ");
scanf("%d", &years);
float total = principal;
for (int i = 1; i <= years; i++) {
total *= (1 + interest);
}
printf("The total amount of principal after %d years is %.2f\n", years, total);
return 0;
}
```
该程序要求用户输入本金金额、利率和存款年数,然后使用一个for循环计算本金合计。在每一年结束时,本金乘以1加上利率的倍数,然后将结果存储在变量total中。最后,程序输出本金合计的总金额。
注意,此程序假定利率为固定值,而实际上利率可能会随时间变化。此外,此程序还没有考虑到复利的影响。
阅读全文
相关推荐






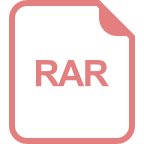



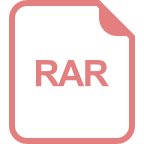
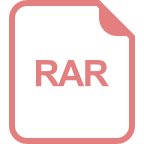



