一个快餐厅有4 类职员:( l )领班:接受顾客点菜;( 2 )厨师:准备顾客的饭菜;( 3 ) 包工:将做好的饭菜打包;( 4 )出纳员:收款并提交食品。每个职员可被看作一个进程,试用一种同步机制写出能让四类职员正确并发运行的程序
时间: 2024-10-14 08:13:04 浏览: 54
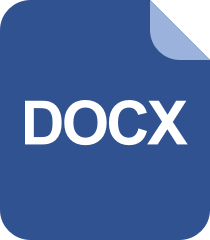
星际顾客定位
在一个快餐店环境中,我们可以使用互斥量(mutex)、信号量(semaphore)或者条件变量(condition variable)等同步机制来协调四个职员(进程)的并发操作。这里提供一个基于条件变量的基本示例,假设我们有一个全局的资源池(例如食物准备区):
```c++
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
class Staff {
public:
enum Type {LEADER, COOK, PACKAGER, CASHIER};
Staff(Type type) : type_(type), foodReady_(false) {}
private:
void ServeFood() {
// 模拟工作过程...
std::this_thread::sleep_for(std::chrono::seconds(2)); // 假设烹饪需要时间
foodReady_ = true;
notifyCook();
}
void Cook() {
while (!foodReady_) {
std::unique_lock<std::mutex> lock(mutex_);
conditionVariable_.wait(lock);
}
// 准备食物...
std::cout << "Chef prepares food for " << type_ << "\n";
foodReady_ = false; // 食物准备完毕,释放资源
}
void PackFood() {
// 模拟包装过程...
std::cout << "Packager packs food for " << type_ << "\n";
}
void Cashier() {
// 模拟收银过程...
std::cout << "Cashier collects payment from " << type_ << "\n";
}
void notifyCook() {
std::lock_guard<std::mutex> lock(mutex_);
foodReady_ = true;
conditionVariable_.notify_one(); // 唤醒等待的厨师
}
const Type type_;
bool foodReady_; // 表示是否有食物准备好
mutable std::mutex mutex_; // 保护共享状态
std::condition_variable conditionVariable_; // 用于同步
};
int main() {
Staff leader("Leader");
Staff cook("Cook"), packager("Packager"), cashier("Cashier");
std::thread t1(leader.ServeFood);
std::thread t2(cook.Cook);
std::thread t3(packager.PackFood);
std::thread t4(cashier.Cashier);
t1.join();
t2.join();
t3.join();
t4.join();
return 0;
}
```
在这个例子中,领班先服务顾客并通知厨师准备食物。当厨师准备好食物时,他们会唤醒正在等待的厨师。同时,其他职员(如包工和出纳员)可以继续自己的任务。这种设计避免了数据竞争,确保了并发工作的正确性。
阅读全文
相关推荐
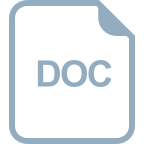
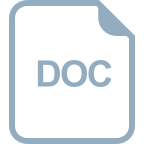
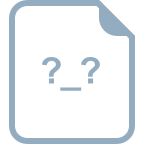
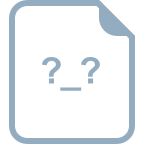
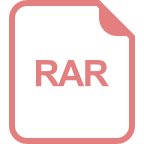
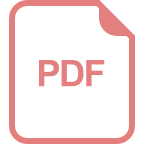
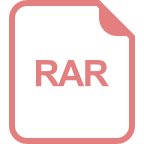
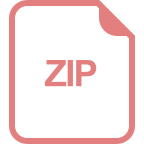
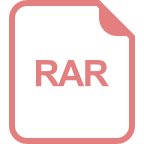
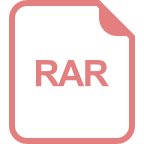
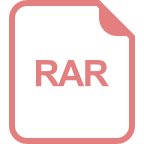
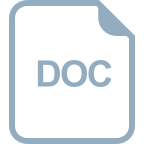
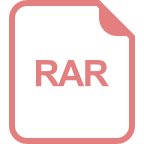
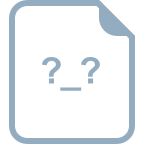
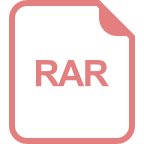
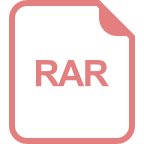
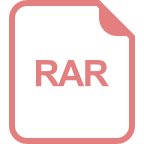
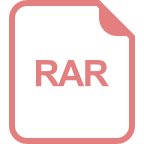