用八种Python方式生成30以内的所有奇数的代码
时间: 2024-09-26 08:11:44 浏览: 39
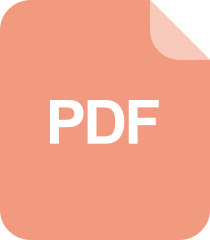
Python-while 计算100以内奇数和的方法

在Python中,有多种方法可以生成30以内的所有奇数。以下是八种常见的实现方式:
1. 列表推导式:
```python
odd_numbers = [x for x in range(1, 31) if x % 2 != 0]
```
2. 传统for循环:
```python
odd_numbers = []
for i in range(1, 31, 2):
odd_numbers.append(i)
```
3. 使用range()函数结合步长:
```python
odd_numbers = list(range(1, 31, 2))
```
4. 使用filter()函数:
```python
from itertools import filterfalse
odd_numbers = list(filterfalse(lambda x: x % 2 == 0, range(1, 31)))
```
5. map()函数配合lambda表达式:
```python
odd_numbers = list(map(lambda x: x, filter(lambda x: x % 2 != 0, range(1, 31))))
```
6. 生成器表达式:
```python
odd_numbers = (x for x in range(1, 31) if x % 2 != 0)
# 若要转换为列表,可以加上list()
# odd_numbers = list(odd_numbers)
```
7. 使用while循环:
```python
odd_numbers = []
i = 1
while i < 31:
odd_numbers.append(i)
i += 2
```
8. 使用enumerate并判断索引和值:
```python
odd_numbers = [num for index, num in enumerate(range(1, 31)) if index % 2 != 0]
```
阅读全文
相关推荐
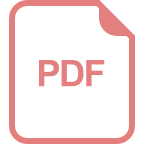
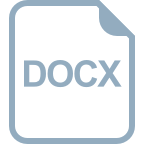















