springboot+定时任务+第三方接口调用Post方式(json参数)
时间: 2024-11-27 19:09:55 浏览: 16
在Spring Boot项目中集成定时任务并调用第三方API通常需要以下几个步骤:
1. **添加依赖**:首先在项目的pom.xml文件中添加Spring Cloud Task、Spring Web以及对HTTP客户端支持的依赖,如`spring-boot-starter-web`和`httpclient`。
```xml
<dependencies>
<!-- ... -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-task</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<!-- ... -->
</dependencies>
```
2. **创建定时任务**:通过`@Scheduled`注解创建一个定时的任务类,该类可以是一个@Component或@Service,包含一个方法用于发送POST请求。
```java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.web.client.RestTemplate;
public class ThirdPartyTask {
private RestTemplate restTemplate = new RestTemplate();
@Scheduled(cron = "0 0/5 * * * ?") // 每隔5分钟执行一次
public void callThirdPartyApi() {
String apiUrl = "https://api.example.com/endpoint";
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
HttpEntity<String> entity = new HttpEntity<>("YourJsonPayload", headers);
try {
ResponseEntity<String> response = restTemplate.postForEntity(apiUrl, entity, String.class);
// 处理响应结果
} catch (Exception e) {
// 处理异常
}
}
}
```
3. **处理异常和错误处理**:在调用第三方API时可能会遇到网络问题或其他异常,记得捕获并处理异常,同时可以设置合理的超时时间和重试策略。
4. **配置启动类**:如果你使用的是Spring Boot Actuator,可以在启动类上启用定时任务功能,并指定定时任务注册中心。
```java
@SpringBootApplication
@EnableScheduling
public class AppApplication {
public static void main(String[] args) {
SpringApplication.run(AppApplication.class, args);
}
}
```
阅读全文
相关推荐
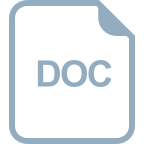
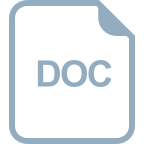
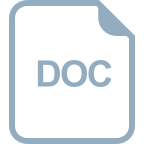
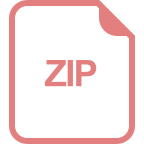
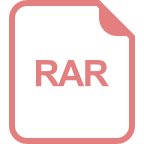
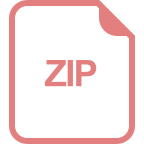
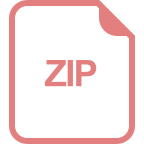
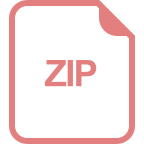
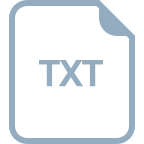
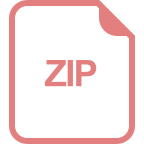
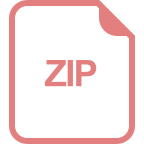
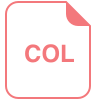

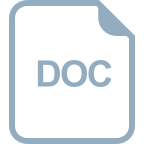
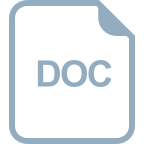
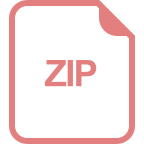
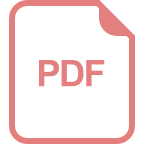
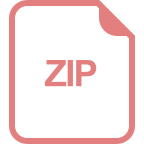
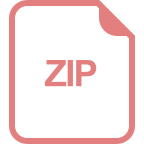