你用一句话来写,而且我不是要去除’,是要去除’s
时间: 2024-09-18 11:12:28 浏览: 59
明白了,您的意思是不需要简单地删除所有的撇号('),而是只针对那些表示所属关系的复数形式的撇号,比如"cats's"应该变成"cats"。这种情况通常涉及到英语中的一些特殊语法,比如省略了"of"的双重所有格。在编程中,可以修改上面提到的正则表达式,只匹配以's'结尾的复数名词形式,然后做相应的替换。具体的实现可能会依赖于特定的词典或规则检查。
如果使用Python,可以尝试基于词典或特定规则来判断是否需要移除's',但这可能需要更复杂的算法:
```python
def maybe_remove_apostrophe(word):
if word.endswith("'s") and not some_check_for_plural_or_special_case(word): # 假设some_check_for_plural_or_special_case是一个辅助函数
word = word[:-2] # 去掉's'
return word
# 示例用法
text = "Each cats's house is cozy."
cleaned_text = ' '.join(maybe_remove_apostrophe(word) for word in text.split())
print(cleaned_text) # 输出: Each cats house is cozy.
```
在这个示例中,`some_check_for_plural_or_special_case()`函数需要你自己根据实际需求设计规则。
相关问题
c语言一句话中去掉多余空格
在 C 语言中,去除字符串中的多余空格通常需要遍历整个字符串,遇到非空格字符就添加到新字符串中。可以使用一个简单的循环结构配合条件判断来实现,例如:
```c
#include <stdio.h>
#include <string.h>
void remove_spaces(char *str) {
if (str != NULL) {
int i = 0, j;
for (j = 0; str[j] != '\0'; ++j) {
if (str[j] != ' ') {
str[i++] = str[j];
}
}
str[i] = '\0'; // 将最后一位非空格字符后面的'\0'也移动到正确位置
} else {
printf("输入的字符串为空!\n");
}
}
int main() {
char input[] = "hello world!";
remove_spaces(input);
printf("%s\n", input); // 输出: hello world!
return 0;
}
```
c语言完成,并且禁止使用ctype.h库函数: 编写程序,输入的一句话,统计里面每个英文字母的出现次数(不区分大小写),对里面输入的 其他字符不进行统计,最后按大写字母顺序统计出每个字母的出现次数。 输入样例: Which question do you like? 1, 2 or 3? 输出样例: C:1 D:1 E:2 H:2 I:3 K:1 L:1 N:1 0:4 Q:1 R:1 S:1 T:1 U :2 W:1 Y:1
以下是完成上述任务的 C 语言代码示例:
#include <stdio.h>
#include <string.h>
#define MAX_LEN 1000 // 定义一句话的最大长度
#define ASCII_a 97 // ASCII 码中小写字母 'a' 的值
#define ASCII_A 65 // ASCII 码中大写字母 'A' 的值
int main()
{
char sentence[MAX_LEN]; // 定义一个字符数组来存储输入的一句话
int alphabet_count[26] = {0}; // 定义一个整型数组来存储每个字母的出现次数,初始值都设为 0
int i, len;
// 输入一句话
printf("请输入一句话:");
fgets(sentence, MAX_LEN, stdin);
// 去除字符串末尾的换行符
len = strlen(sentence);
if (sentence[len - 1] == '\n') {
sentence[len - 1] = '\0';
}
// 统计每个字母的出现次数
for (i = 0; i < len; i++) {
// 如果当前字符是小写字母
if (sentence[i] >= 'a' && sentence[i] <= 'z') {
alphabet_count[sentence[i] - ASCII_a]++;
}
// 如果当前字符是大写字母
else if (sentence[i] >= 'A' && sentence[i] <= 'Z') {
alphabet_count[sentence[i] - ASCII_A]++;
}
}
// 按大写字母顺序输出每个字母的出现次数
for (i = 0; i < 26; i++) {
printf("%c:%d ", 'A' + i, alphabet_count[i]);
}
return 0;
}
上述代码的工作流程如下:
1. 先输入一句话,存储在字符数组 sentence 中。
2. 去除字符串末
阅读全文
相关推荐
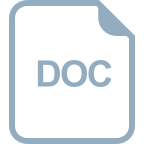
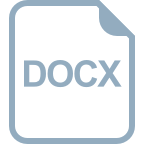
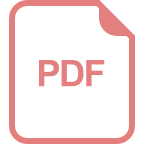
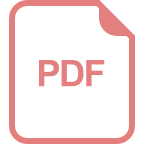
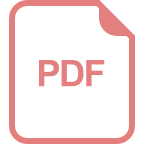
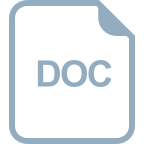
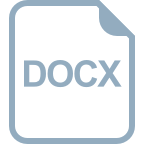






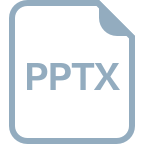
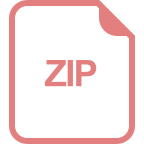