从文件course.txt 读取课程信息,放入线性表L中,直接在线性表表尾插入,返回值为读取的课程信息数c语言代码
时间: 2024-10-19 09:11:24 浏览: 42
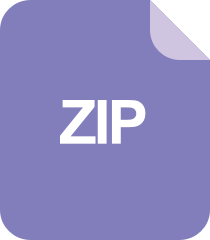
基于C语言实现线性表的建立、插入、删除、查找等基本操作.zip
在C语言中,你可以使用`fopen()`函数打开文件,`fgets()`读取文件内容,然后使用字符串处理功能解析输入,最后将数据插入到链表的尾部。这里假设你已经有一个简单的字符数组作为链表节点。以下是基本的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 链表节点结构
typedef struct CourseNode {
char course[50]; // 假设每个课程名称最多50个字符
struct CourseNode *next;
} CourseNode;
// 初始化一个空链表
CourseNode* createList() {
return NULL;
}
// 插入课程到链表尾部
void insertCourse(CourseNode** head, const char* course) {
CourseNode* newNode = (CourseNode*)malloc(sizeof(CourseNode));
strncpy(newNode->course, course, sizeof(newNode->course) - 1); // 注意不要包含'\0'
newNode->next = NULL;
if(*head == NULL) {
*head = newNode;
} else {
CourseNode* temp = *head;
while(temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
int readCoursesFromFile(const char* filename, CourseNode** head) {
int count = 0;
FILE* file = fopen(filename, "r");
if(file == NULL) {
printf("Error opening the file.\n");
return 0;
}
CourseNode* newNode = createList();
char line[100];
while(fgets(line, sizeof(line), file)) { // 读取每行
if(line[0] != '#' && line[0] != '\n') { // 忽略注释和空行
insertCourse(head, line);
count++;
}
}
fclose(file);
return count;
}
int main() {
CourseNode* head = NULL;
int coursesRead = readCoursesFromFile("course.txt", &head);
printf("Read %d courses into the list.\n", coursesRead);
return 0;
}
```
注意:这段代码只是一个基本的示例,实际操作中需要处理可能出现的错误,如文件打开失败、内存分配失败等情况。此外,如果课程名中包含换行符或其他特殊字符,可能需要额外处理。
阅读全文
相关推荐
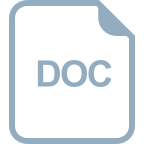
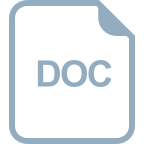
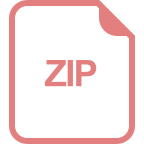
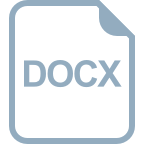
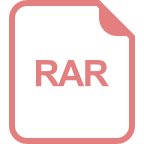
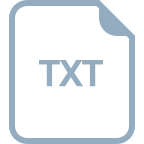
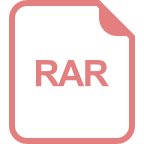
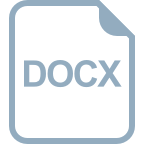
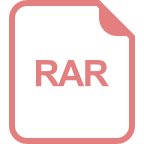
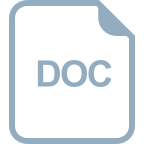
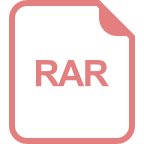
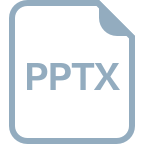
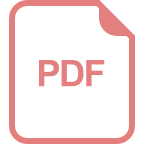
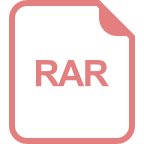
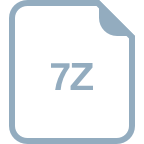