python 读取文件 写入字典
时间: 2024-11-20 20:24:15 浏览: 31
在Python中,你可以使用内置的`open()`函数和文件操作来读取文件内容并将其转换为字典。这里是一个基本的例子:
```python
# 打开文件,如果不存在则创建(默认模式是'r', 可以选择其他如'a'追加)
with open('file.txt', 'r') as file:
# 读取文件的每一行,假设每行数据是以逗号分隔的键值对
lines = file.read().splitlines()
# 创建空字典
data_dict = {}
# 遍历每一行,将每一对分割的值作为键值添加到字典中
for line in lines:
key, value = line.split(',')
data_dict[key] = value
# 现在data_dict包含了从文件中读取的内容
print(data_dict)
# 如果需要写入字典回文件,可以这样做:
with open('file.txt', 'w') as file:
for key, value in data_dict.items():
file.write(f'{key},{value}\n')
```
在这个例子中,我们假设文件内容是文本格式,每行有一个键值对,通过`read()`获取所有行,然后逐行处理。写入文件时,同样遍历字典,每个键值对写成一行。
相关问题
在Python中如何写入字典到文件,然后再读取到列表? 在Python中如何写入字典到文件,然后再读取到列表? 在Python中如何不使用第三方模块写入字典到文件,然后再读取到列表?
在Python中,如果不使用第三方模块,可以直接通过内置的`pickle`模块来操作二进制数据,将字典序列化到文件,然后再反序列化回字典列表。以下是步骤:
1. 写入字典到文件:
```python
import pickle
# 假设有一个字典列表
data_list = [{"name": "Alice", "age": 20}, {"name": "Bob", "age": 25}]
# 打开一个二进制文件模式(wb表示write binary)
with open("data.pkl", "wb") as f:
# 使用pickle.dump函数将字典列表写入文件
pickle.dump(data_list, f)
```
2. 从文件中读取字典列表:
```python
# 打开二进制文件模式(rb表示read binary)
with open("data.pkl", "rb") as f:
# 使用pickle.load函数从文件中加载数据,返回的是原始的对象形式,可能是元组、列表或字典,这里仍然是一个列表
loaded_list = pickle.load(f)
```
由于`pickle`是非标准格式,所以它不支持直接转为列表,但在内存里,它依然是一个列表对象。
python将字典写入文件以及从文件中读取字典数据
以下是Python将字典写入文件以及从文件中读取字典数据的示例:
1. 将字典写入文件:
```python
import json
data = {"name": "John", "age": 30, "city": "New York"}
with open("data.json", "w") as file:
json.dump(data, file)
```
2. 从文件中读取字典数据:
```python
import json
with open("data.json", "r") as file:
data = json.load(file)
print(data)
```
阅读全文
相关推荐
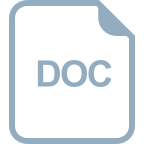
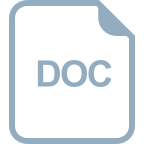
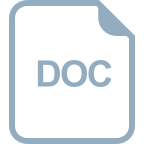
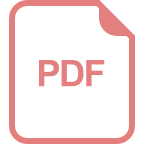
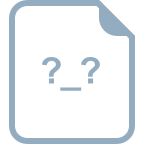
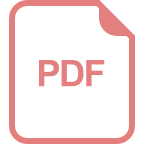
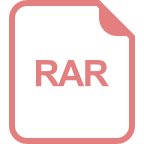
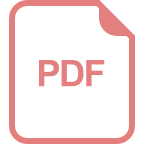
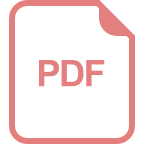
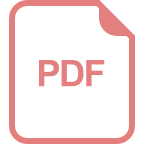
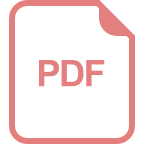
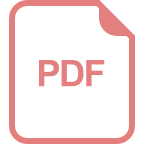
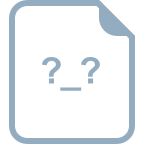
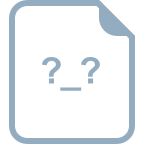
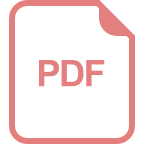
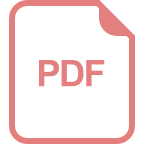
