stem layer
时间: 2023-11-15 17:58:00 浏览: 706
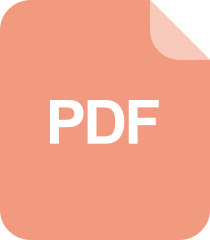
中国STEM 教育白皮书
Stem layer是卷积神经网络(CNN)的骨干层,其主要目的是降低计算复杂度。在CNN中,输入图像首先通过stem layer进行卷积和池化操作,然后再进入后续的卷积层进行特征提取和分类。stem layer通常包括一些卷积层和池化层,用于对输入图像进行初步的特征提取和降维,以减少后续卷积层的计算量。通过使用stem layer,可以有效地降低CNN的计算复杂度,提高模型的训练速度和准确率。
阅读全文
相关推荐
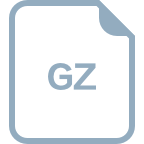
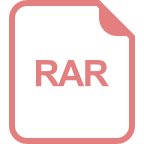



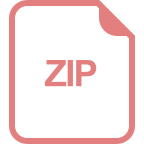
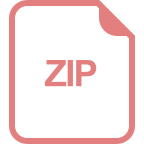
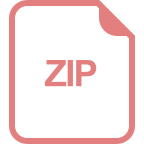
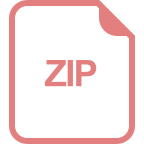
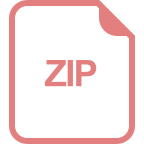
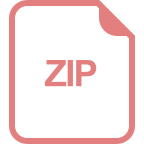
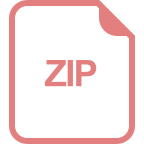
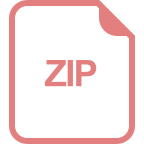
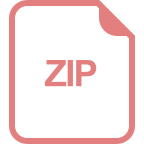
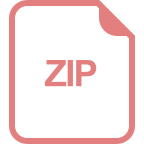
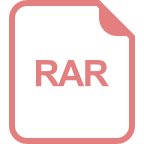
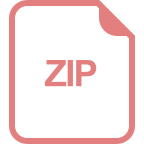