#include<iostream> #include<queue> #include<cstring> using namespace std; int map[1000][1000]; int step[1000][1000]; int stepx[10]={0,0,-1,-1}; int stepy[10]={-1,1,0,0}; int n,m; queue<int> qx,qy; int x0,y0,x1,y1,x2,y2; int bfs() { memset(step,-1,sizeof(step)); step[x0][y0]=0; qx.push(x0); qy.push(y0); while(qx.size()) { int tx=qx.front(); int ty=qy.front(); qx.pop(); qy.pop(); for(int i=0;i<4;++i) { x2=tx+stepx[i]; y2=ty+stepy[i]; if(map[x2][y2]==0||x2<1||x2>n||y2<1||y2>m||step[x2][y2]!=-1)continue; qx.push(x2); qy.push(y2); step[x2][y2]=step[tx][ty]+1; } } return step[x1][y1]; } int main() { cin>>n; cin>>m; for(int i=1;i<=n;++i) for(int j=1;j<=m;++j) cin>>map[i][j]; cin>>x0>>y0>>x1>>y1; cout<<bfs()<<endl; for(int i=1;i<=n;++i) { for(int j=1;j<=m;++j) cout<<step[i][j]<<" "; cout<<endl; } return 0; }有什么错误
时间: 2024-04-11 07:27:14 浏览: 26
根据您提供的代码,有几个问题需要解决:
1. 在使用`map`作为数组名时,可能会与`map`函数发生冲突,导致编译错误。为了避免这种冲突,您可以将数组名更改为其他名称。
2. 在使用`memset`函数时,第一个参数应为指针类型。因此,您需要将`step`数组作为参数传递给`memset`函数时取其地址。
修改后的代码如下:
```cpp
#include<iostream>
#include<queue>
#include<cstring>
using namespace std;
int myMap[1000][1000];
int step[1000][1000];
int stepx[10]={0,0,-1,-1};
int stepy[10]={-1,1,0,0};
int n,m;
queue<int> qx,qy;
int x0,y0,x1,y1,x2,y2;
int bfs()
{
memset(&step,-1,sizeof(step));
step[x0][y0]=0;
qx.push(x0);
qy.push(y0);
while(qx.size())
{
int tx=qx.front();
int ty=qy.front();
qx.pop();
qy.pop();
for(int i=0;i<4;++i)
{
x2=tx+stepx[i];
y2=ty+stepy[i];
if(myMap[x2][y2]==0||x2<1||x2>n||y2<1||y2>m||step[x2][y2]!=-1)continue;
qx.push(x2);
qy.push(y2);
step[x2][y2]=step[tx][ty]+1;
}
}
return step[x1][y1];
}
int main()
{
cin>>n;
cin>>m;
for(int i=1;i<=n;++i)
for(int j=1;j<=m;++j)
cin>>myMap[i][j];
cin>>x0>>y0>>x1>>y1;
cout<<bfs()<<endl;
for(int i=1;i<=n;++i)
{
for(int j=1;j<=m;++j)
cout<<step[i][j]<<" ";
cout<<endl;
}
return 0;
}
```
这样修改后的代码应该可以解决编译错误。请注意,这里只是解决了代码中的一些错误,如果还有其他问题,您可以提供更多的细节或错误信息。
相关推荐
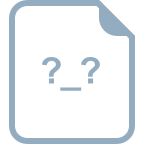















