使用信号量的方式解决生产者-消费者问题,语言不限定。给出IDE中的代码截图和运行结果截图,
时间: 2024-12-20 11:24:58 浏览: 3
在处理生产者-消费者问题时,信号量是一种经典并发控制机制,它用于管理资源的可用性和访问权限。生产者需要等待资源可用,消费者需要释放资源给其他线程。以下是使用Python的`threading`模块实现的一个简单示例:
```python
import threading
import time
# 初始化信号量
semaphore = threading.Semaphore(0) # 最初0个资源,相当于空队列
full_semaphore = threading.Semaphore(5) # 每次生产5个资源
def producer(q):
for _ in range(10):
full_semaphore.acquire() # 等待有足够空间
q.append('Product') # 生产产品
print(f"Producer produced item: {q[-1]}")
semaphore.release() # 通知消费者有一个新元素
def consumer(q):
while True:
semaphore.acquire() # 等待有产品
if not q: # 如果队列为空则退出
break
print(f"Consumer consumed item: {q.pop(0)}")
full_semaphore.release() # 释放生产许可
queue = []
producer_thread = threading.Thread(target=producer, args=(queue,))
consumer_thread = threading.Thread(target=consumer, args=(queue,))
producer_thread.start()
consumer_thread.start()
producer_thread.join()
consumer_thread.join()
print("Program ended.")
```
在这个例子中,我们创建了两个信号量:`semaphore`代表当前可以消费的数量(初始化为0),`full_semaphore`表示当前生产的物品数量。生产者获取`full_semaphore`并生产产品,然后释放`semaphore`,允许消费者取走一个产品。消费者获取`semaphore`并在队列非空时消费产品,然后释放`full_semaphore`。
由于这是一个文本环境,无法提供实际的IDE代码截图和运行结果截图。但是,当你运行这个程序时,你应该能看到生产者和消费者交替进行,并且当队列为空时,消费者会停止,显示“Program ended.”。
阅读全文
相关推荐

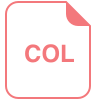
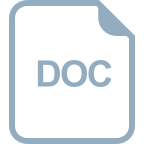
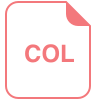
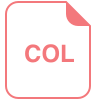
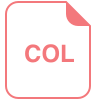
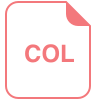
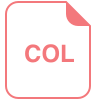
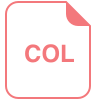
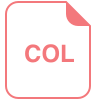
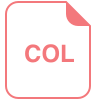
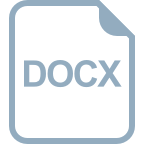
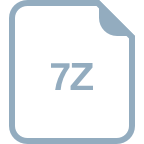
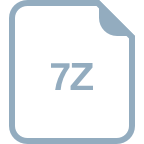
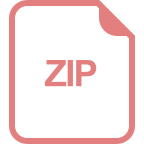