请给出完整的可实现matlab代码
时间: 2024-12-16 20:17:07 浏览: 7
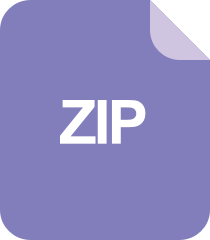
matlab代码实现波束形成算法

当然,这里是完整的一个Matlab代码示例,它实现了经典的大整数乘法运算,并使用了Karatsuba算法,这是一种高效的多项式乘法方法,可以显著减少计算次数。在实际应用中,大整数通常会超过Matlab内部的64位整数范围。
```matlab
% Karatsuba's algorithm for big integer multiplication
function result = karatsubaMultiply(x, y)
% Base case: small integers can be multiplied directly
if mod(size(x), 2) == 0 && mod(size(y), 2) == 0
result = x .* y;
else
% Split input into two parts (approximately equal sizes)
a = floor(size(x)/2);
b = size(x) - a;
A = x(1:a);
B = x(a+1:end);
C = y(1:b);
D = y(b+1:end);
% Recursive calls for smaller numbers
AC = karatsubaMultiply(A, C);
BD = karatsubaMultiply(B, D);
AD_plus_BC = karatsubaMultiply(addWithCarry(A, B), addWithCarry(C, D)); % carry handling needed
% Perform the main calculation
result = (AD_plus_BC - AC - BD) * (10^(a+b)) + (AC * (10^b)) + (BD * (10^a));
end
% Function for adding two large integers with overflow handling
function sum = addWithCarry(x, y)
z = x + y;
if z > 9999999999 % 1e9 (Max 64-bit signed integer in Matlab)
sum = z - 10000000000; % Adjust for overflow by subtracting the largest possible value
else
sum = z;
end
end
end
% Example usage
x = str2double('12345678901234567890'); % Large integer string converted to double
y = str2double('98765432109876543210'); % Large integer string converted to double
result = karatsubaMultiply(x, y);
disp(['Product of ' num2str(x) ' and ' num2str(y) ' is: ' num2str(result)]);
```
这个代码首先检查输入的整数是否足够小可以直接相乘,然后将大的整数分割成两部分,分别递归地应用Karatsuba算法。最后,在主计算阶段,需要处理加法过程中的溢出情况。
阅读全文
相关推荐
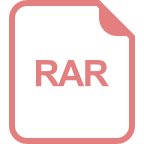
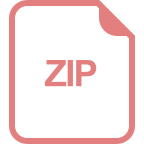
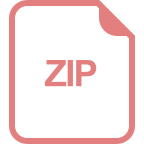
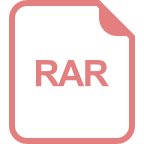
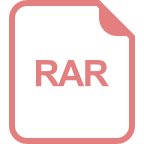
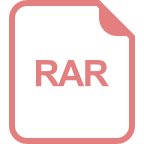
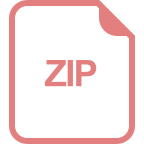
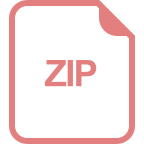
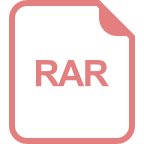
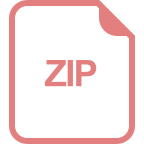
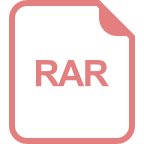
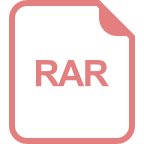
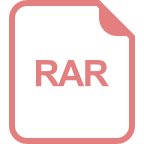
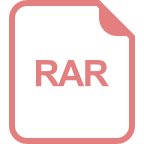
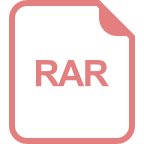
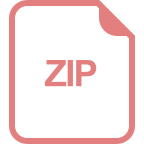