excel 导入导出 java 例子
时间: 2023-11-23 22:03:29 浏览: 85
在Java中,我们可以使用Apache POI库来实现Excel的导入和导出。下面是一个简单的例子来展示如何在Java中实现Excel的导入和导出功能。
Excel导出:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelExportExample {
public static void main(String[] args) {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("Name");
headerRow.createCell(1).setCellValue("Age");
headerRow.createCell(2).setCellValue("Location");
Row dataRow = sheet.createRow(1);
dataRow.createCell(0).setCellValue("John");
dataRow.createCell(1).setCellValue(25);
dataRow.createCell(2).setCellValue("New York");
try {
FileOutputStream fileOut = new FileOutputStream("example.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
Excel导入:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelImportExample {
public static void main(String[] args) {
try {
FileInputStream file = new FileInputStream(new File("example.xlsx"));
Workbook workbook = new XSSFWorkbook(file);
Sheet sheet = workbook.getSheetAt(0);
Row row = sheet.getRow(1);
Cell cell1 = row.getCell(0);
Cell cell2 = row.getCell(1);
Cell cell3 = row.getCell(2);
System.out.println("Name: " + cell1.getStringCellValue());
System.out.println("Age: " + (int) cell2.getNumericCellValue());
System.out.println("Location: " + cell3.getStringCellValue());
workbook.close();
file.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上是一个简单的例子来展示如何在Java中实现Excel的导入和导出功能,我们可以根据具体的需求进行进一步的扩展和定制化。
阅读全文
相关推荐
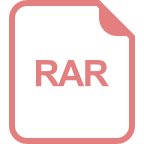
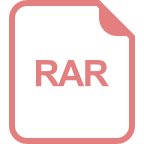
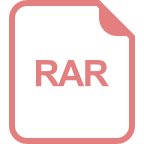
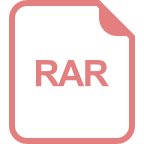
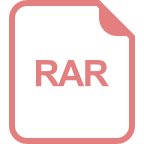
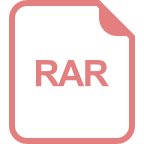
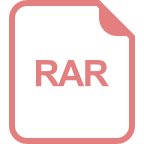
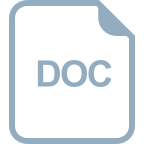
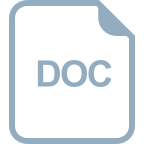
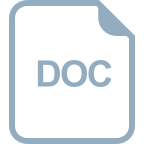
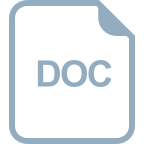
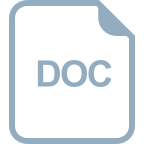
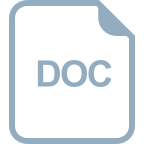


