python拷贝图片到指定文件夹
时间: 2024-10-05 08:02:15 浏览: 27
在Python中,你可以使用`PIL`(Python Imaging Library)库来处理图片,然后利用`shutil`库的`copyfile()`函数将图片复制到特定的文件夹。这里是一个简单的示例:
```python
from PIL import Image
import shutil
# 打开图片文件
img = Image.open('原图路径.jpg')
# 指定目标文件夹路径
destination_folder = '目标文件夹路径'
# 如果目标文件夹不存在,创建它
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
# 新建图片名称(这里可以保留原名,也可以自定义)
new_image_name = '新图片名称.jpg'
# 完整的复制路径
full_destination_path = os.path.join(destination_folder, new_image_name)
# 复制图片
shutil.copyfile(img.filename, full_destination_path)
```
在这个例子中,你需要替换`原图路径.jpg`为你想要复制的图片的实际路径,以及`目标文件夹路径`为目标文件夹的位置。
相关问题
python2拷贝指定文件到指定文件夹内
可以使用 `shutil` 模块来实现拷贝指定文件到指定文件夹内的功能。具体代码如下:
```python
import shutil
# 拷贝文件
shutil.copy('path/to/source/file', 'path/to/destination/folder/')
```
其中,`path/to/source/file` 是需要拷贝的文件路径,`path/to/destination/folder/` 是目标文件夹路径,需要以 `/` 结尾。
如果需要拷贝多个文件,可以使用 `os.listdir()` 函数列出指定文件夹内的所有文件,然后对每个文件进行拷贝操作。具体代码如下:
```python
import os
import shutil
# 列出指定文件夹内的所有文件
files = os.listdir('path/to/source/folder/')
# 拷贝每个文件到目标文件夹内
for file in files:
shutil.copy('path/to/source/folder/' + file, 'path/to/destination/folder/')
```
其中,`path/to/source/folder/` 是需要拷贝文件的文件夹路径,`path/to/destination/folder/` 是目标文件夹路径,需要以 `/` 结尾。拷贝时需要使用文件夹路径和文件名拼接成完整的文件路径。
python拷贝文件夹中的文件
你可以使用shutil模块中的`copytree()`函数来拷贝文件夹中的文件。下面是一个示例代码:
```python
import shutil
# 指定源文件夹和目标文件夹路径
src_folder = "/path/to/source/folder"
dst_folder = "/path/to/destination/folder"
# 使用shutil模块中的copytree()函数拷贝文件夹
shutil.copytree(src_folder, dst_folder)
```
这个代码会将源文件夹中的所有文件和文件夹拷贝到目标文件夹中。如果目标文件夹已经存在,则会抛出`FileExistsError`异常。如果你需要覆盖目标文件夹中的文件,可以使用`shutil.rmtree()`函数删除目标文件夹,然后再执行拷贝操作。
相关推荐
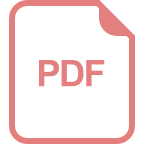
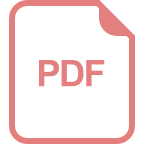
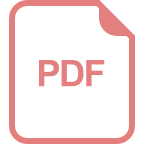
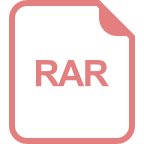
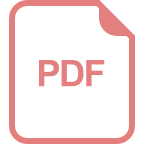
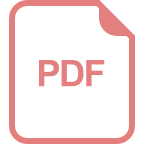
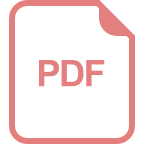
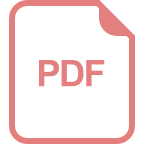
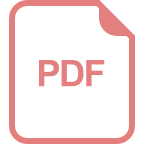
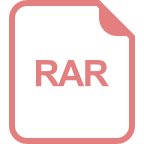
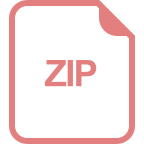



