编写学生基本信息管理程序。用链表存储一个班的学生信息(最多100位学生),每个学生的信息有:学号,姓名,性别,联系电话和家庭住址。需要实现的功能有:新建学生信息链表,对学生信息进行增加、删除和查找。
时间: 2024-09-12 12:08:19 浏览: 94
学生基本信息管理程序是一个简单的数据管理系统,它通过链表结构来存储和管理学生信息。链表是一种常见的数据结构,它由一系列节点组成,每个节点包含数据部分和指向下一个节点的指针。在本例中,每个节点将存储一位学生的信息。
首先,我们需要定义一个学生信息的数据结构,如下所示:
```c
typedef struct Student {
char studentID[20]; // 学号
char name[50]; // 姓名
char gender[10]; // 性别
char phone[20]; // 联系电话
char address[100]; // 家庭住址
struct Student *next; // 指向下一个学生信息的指针
} Student;
```
然后,我们需要初始化链表,创建一个头节点,它不存储学生信息,仅作为链表的起始点:
```c
Student *head = NULL;
```
接下来,我们将实现以下几个功能:
1. **新建学生信息链表**:
- 初始化时创建头节点,链表为空。
```c
head = (Student *)malloc(sizeof(Student));
if (head != NULL) {
head->next = NULL;
}
```
2. **增加学生信息**:
- 创建一个新的学生节点,并要求用户输入学生信息。
- 将新节点插入到链表的末尾。
```c
void AddStudent(Student **head) {
Student *newStudent = (Student *)malloc(sizeof(Student));
if (newStudent != NULL) {
// 输入学生信息代码...
// 假设有一个函数 InputStudentInfo() 用于输入信息
InputStudentInfo(newStudent);
// 插入到链表末尾
newStudent->next = NULL;
if (*head == NULL) {
*head = newStudent;
} else {
Student *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newStudent;
}
}
}
```
3. **删除学生信息**:
- 根据学号查找学生节点,并删除。
- 删除时需要维护好链表的连续性,防止内存泄漏。
```c
void DeleteStudent(Student **head, const char *studentID) {
Student *current = *head;
Student *previous = NULL;
while (current != NULL && strcmp(current->studentID, studentID) != 0) {
previous = current;
current = current->next;
}
if (current != NULL) {
if (previous == NULL) {
// 删除的是头节点
*head = current->next;
} else {
previous->next = current->next;
}
free(current);
}
}
```
4. **查找学生信息**:
- 根据学号来查找特定的学生节点。
```c
Student *FindStudent(Student *head, const char *studentID) {
Student *current = head;
while (current != NULL) {
if (strcmp(current->studentID, studentID) == 0) {
return current;
}
current = current->next;
}
return NULL;
}
```
以上是基本的数据结构设计和几个主要功能的实现方法。实现时还需要注意输入验证、内存管理等问题。
阅读全文
相关推荐
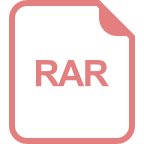















