revit开发一个插件,创建一个嵌套族,给出完整代码
时间: 2024-12-22 07:32:22 浏览: 9
Revit API提供了一个强大的框架来开发自定义插件,包括创建嵌套族的功能。在Revit中,嵌套族(Nested Family)是指可以在另一个族中使用的子族。以下是一个简单的例子,展示了如何使用C#编写一个基础的Revit插件来创建嵌套族:
```csharp
using Autodesk.Revit.DB;
using Autodesk.Revit.UI;
[Transaction(TransactionMode.ReadOnly)]
public class CreateNestedFamily : IExternalCommand
{
public Result Execute(ExternalCommandData commandData, ref string message, ElementSet elements)
{
try
{
// 获取当前项目
Document doc = commandData.Application.ActiveUIDocument.Document;
// 创建新的嵌套族类型
FamilyType parentType = doc.GetElement(commandData.InputParameters[0].AsElementId()).AsFamilyType();
Family newNestedType = doc.FamilyCreate.NewFamilyType(parentType);
// 添加族参数
FamilyParameter param = new NestedParamDefinition(newNestedType);
newNestedType.Parameters.Add(param);
// 创建嵌套族实例
FamilyInstance nestedInstance = newNestedType.CreateNewInstance(doc);
// 将嵌套族添加到父族中
parentType.GetNestedFamilyTypes().Add(nestedInstance.GetType());
message = "Nested family created successfully.";
return Result.Succeeded;
}
catch (Exception ex)
{
message = $"Error: {ex.Message}";
return Result.Failed;
}
}
private FamilyParameter NestedParamDefinition(FamilyType type)
{
// 这里只是一个简单的参数定义示例,你可以根据需要设置参数类型、名称等
BuiltInParameter categoryParam = BuiltInParameter.PROJECT_NAME_PARAM;
Category defaultCategory = Category.GetCategory(doc, BuiltInCategory.OST_GenericModel);
return type.AddParameter("Nested Param", "A parameter for the nested family.", categoryParam, defaultCategory);
}
}
// 插件注册
[Autodesk.Revit.UI.AddInRegistration]
class AddInRegistration
{
[Autodesk.Revit.UI.AddInMenu("Custom Menu", "My Tools")]
public static readonly CommandId MyCommandId = new CommandId(Guid.Parse("YOUR_ADDIN_GUID"), 1001); // Replace with your addin's GUID
[Autodesk.Revit.UI.Dockable(true)]
public static readonly DockPanelType MyDockPanelType = DockPanelType.Unknown;
[Autodesk.Revit.UI.Transaction]
public static void Initialize()
{
CommandFactory factory = new CommandFactory();
factory.Name = "Create Nested Family";
factory.Id = MyCommandId;
factory.DocumentFilter = DocumentFilterUtils.AllDocuments;
factory.ExecuteMethod = delegate (ExternalCommandData commandData) => new CreateNestedFamily().Execute(commandData, out _, default(ElementSet));
}
}
```
在这个例子中,你需要替换`YOUR_ADDIN_GUID`为你的插件的实际GUID。此外,`NestedParamDefinition`函数可以根据需求定制嵌套家族的参数。
阅读全文
相关推荐
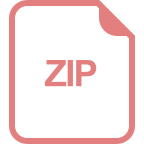
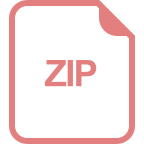
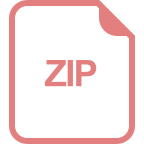




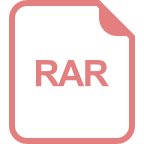
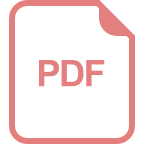
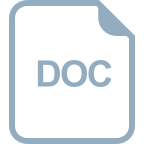







