linux的C语言显示当前目录的文件
时间: 2024-10-01 16:11:48 浏览: 33
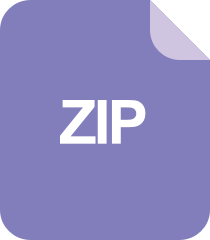
C语言模拟实现Linux文件系统

在Linux系统中,如果你想要使用C语言来显示当前目录下的文件,你可以通过标准库函数`opendir()`, `readdir()`, 和 `closedir()` 来实现。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <dirent.h>
#include <sys/stat.h>
void list_files(char* directory) {
DIR *dir;
struct dirent *entry;
// 打开目录
if ((dir = opendir(directory)) == NULL) {
perror("Error opening directory");
return;
}
// 遍历目录中的每个条目
while ((entry = readdir(dir)) != NULL) {
if (strcmp(entry->d_name, ".") == 0 || strcmp(entry->d_name, "..") == 0) continue; // 忽略"."和".."
struct stat file_stats;
if (stat(entry->d_name, &file_stats) != 0) {
perror("Error getting file stats");
continue;
}
printf("%s - %s\n", entry->d_name, file_stats.st_mode ? "directory" : "file"); // 输出文件名和类型
}
// 关闭目录
closedir(dir);
}
int main() {
char current_dir[PATH_MAX]; // 获取当前工作目录
getcwd(current_dir, PATH_MAX); // 获取并保存当前目录
list_files(current_dir);
return 0;
}
```
在这个程序中,`getcwd()` 函数用于获取当前工作目录,然后`list_files()` 函数会打开这个目录,读取其中的条目,并判断它们是否是文件还是目录。
阅读全文
相关推荐
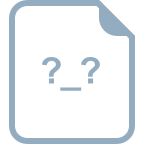
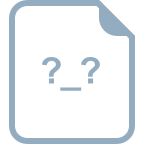
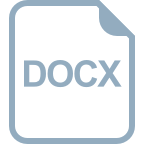
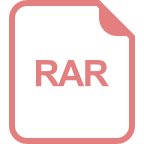
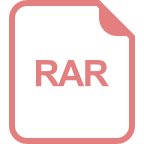
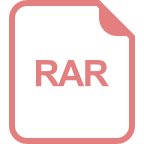
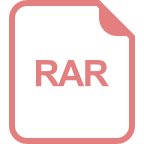
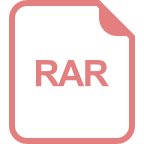
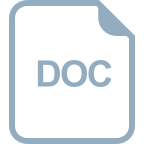
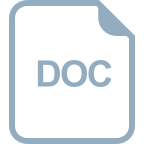



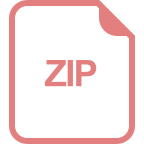