c++获取Mac地址
时间: 2024-09-26 10:18:41 浏览: 15
在 C++ 中,获取计算机的 MAC 地址通常需要访问操作系统的网络接口信息。这通常不是标准库的一部分,而是通过系统调用或者第三方库来实现。以下是一个简单的示例,展示了如何使用 `ifconfig` 命令行工具获取本地网卡的 MAC 地址,但这并不是直接从编程语言层面获取:
```cpp
#include <string>
#include <cstdlib>
std::string getMACAddress() {
std::string command = "ifconfig | grep -o -m 1 'ether [0-9a-f]{1,2}:[0-9a-f]{1,2}:[0-9a-f]{1,2}:[0-9a-f]{1,2}:[0-9a-f]{1,2}:[0-9a-f]{1,2}'";
FILE* pipe = popen(command.c_str(), "r");
if (!pipe) {
throw std::runtime_error("Failed to execute command");
}
char buffer[64];
std::string mac;
while (fgets(buffer, sizeof(buffer), pipe)) {
size_t pos = buffer.find(' ');
if (pos != std::string::npos) {
mac = buffer.substr(5, pos - 5);
break;
}
}
pclose(pipe);
// 将十六进制字符串转换为冒号分隔的格式
return mac.replace(mac.begin(), mac.end(), 2, ":");
}
int main() {
try {
std::cout << "MAC Address: " << getMACAddress() << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
注意这个示例依赖于 `ifconfig` 工具的存在,如果你在 Windows 环境下,可能需要使用 `GetAdaptersInfo` 函数或者第三方库(如 WinPcap 或者 libpcap)。
相关推荐
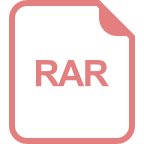
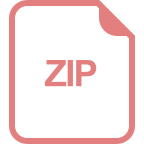
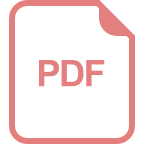














