C语言输入一个整数n判断是否为素数输出yes
时间: 2024-12-17 19:51:20 浏览: 6
在C语言中,你可以通过以下步骤编写一个程序来判断一个给定的整数n是否为素数,并输出相应的结果"yes"或"no":
```c
#include <stdio.h>
// 判断是否为素数的函数
int isPrime(int n) {
if (n <= 1) {
return 0; // 1不是质数,0表示不是
}
for (int i = 2; i * i <= n; i++) { // 只需检查到根号n即可
if (n % i == 0) {
return 0;
}
}
return 1; // 如果没找到因子,则n是质数
}
int main() {
int n;
printf("请输入一个整数: ");
scanf("%d", &n);
if (isPrime(n)) {
printf("yes\n");
} else {
printf("no\n");
}
return 0;
}
```
相关问题
写一段代码C语言输入一个整数,判断其是否是素数,是输出yes,不是输出no。
很高兴回答您的问题!以下是C语言代码:
```
#include <stdio.h>
int main() {
int num;
int isPrime = 1;
printf("请输入一个整数:");
scanf("%d", &num);
if (num <= 1) {
isPrime = 0;
} else {
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) {
isPrime = 0;
break;
}
}
}
if (isPrime) {
printf("yes\n");
} else {
printf("no\n");
}
return 0;
}
```
希望能够帮到您!有其他问题请继续问我哦~
C语言输入一个整数 n,如果n是绝对素数,则输出:Yes, 否则输出:No
在C语言中,你可以编写一个函数来检查一个给定的整数是否是绝对素数(也称为奇异数,是指大于1的自然数,除了1和自身外,没有任何正因数)。这里有一个简单的实现思路:
```c
#include <stdbool.h>
#include <stdio.h>
bool isAbsPrime(int n) {
if (n <= 1) { // Numbers less than or equal to 1 are not considered prime
return false;
}
for (int i = 2; i * i <= n; i++) { // Check up to the square root of n, as factors come in pairs
if (n % i == 0) {
return false; // If n has a factor, it's not an absolute prime
}
}
return true; // If no factors found, n is an absolute prime
}
int main() {
int n;
printf("Enter an integer: ");
scanf("%d", &n);
if (isAbsPrime(n)) {
printf("Yes, ");
} else {
printf("No, ");
}
if (n > 1 && isAbsPrime(n - 2)) { // Additional check to see if n-2 is also a prime
printf("it is also an absolute prime (n-2 is prime)\n");
} else {
printf("\n");
}
return 0;
}
```
在这个程序中,用户输入一个整数,然后我们判断它是否为绝对素数,并输出相应的结果。如果你想要额外确认`n-2`也是绝对素数,我们会做额外的检查。
阅读全文
相关推荐
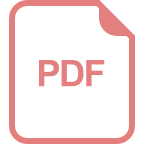
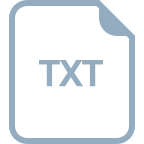
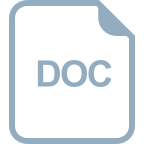













