请用【c语言】帮我写一段代码,计算pi的值,并使用简单的while语句
时间: 2024-12-18 17:15:54 浏览: 23
在C语言中,你可以使用Chudnovsky算法来估算圆周率π,它是一种迭代算法,通过级数的方式来逼近π。这里是一个简单的示例,使用while循环实现:
```c
#include <stdio.h>
#include <math.h>
#define LIMIT 100000 // 级数项的数量
int main() {
double term = 0, pi = 0, denominator = 1;
int n = 0;
while (n < LIMIT) {
term = term + (4 / ((double)(2 * n + 1)) * pow(-1, n));
pi += term;
n++;
denominator *= 6 * n * (n + 1);
}
// 将pi值转换为小数点后6位
pi /= denominator;
pi *= (long long)(1e6); // 四舍五入到百万分之一位
printf("Approximation of Pi using Chudnovsky algorithm: %.6lf\n", pi);
return 0;
}
```
这个程序会打印出Chudnovsky算法估计的π值,注意结果并不精确,随着LIMIT的增加,精度会逐渐提高。如果你想得到更准确的结果,可以适当增加`LIMIT`的值。
相关问题
用c语言编写一段计算圆的周长代码
以下是用C语言编写计算圆的周长的代码:
```c
#include <stdio.h>
int main() {
float radius, circumference;
const float PI = 3.14159265358979323846;
printf("请输入圆的半径:");
scanf("%f", &radius);
circumference = 2 * PI * radius;
printf("圆的周长为:%.2f", circumference);
return 0;
}
```
这段代码的逻辑如下:
1. 引入头文件 `stdio.h`。
2. 在 `main` 函数中定义两个变量,`radius` 和 `circumference`,分别表示圆的半径和周长。另外,定义一个常量 `PI`,表示圆周率。
3. 通过 `printf` 函数向用户询问圆的半径,并通过 `scanf` 函数获取用户输入的半径值。
4. 根据周长的公式 `circumference = 2 * PI * radius` 计算圆的周长。
5. 通过 `printf` 函数将计算出的周长值输出给用户,并使用格式化字符串控制小数位数。
6. `return` 语句表示程序运行结束,返回 0 表示成功执行。
写一段输出圆圈的C语言代码
### 回答1:
#include <stdio.h>
#include <graphics.h> int main()
{
int gd = DETECT, gm;
initgraph(&gd, &gm, NULL);
// 画一个圆圈
circle(250, 250, 50);
getch();
closegraph();
return 0;
}
### 回答2:
下面是一个输出圆圈的C语言代码示例:
```c
#include <stdio.h>
#include <math.h>
int main() {
int radius = 5; // 圆的半径
int centerX = 0; // 圆心的x坐标
int centerY = 0; // 圆心的y坐标
// 循环遍历每一个像素点,判断该点是否在圆内
for (int x = centerX - radius; x <= centerX + radius; x++) {
for (int y = centerY - radius; y <= centerY + radius; y++) {
// 计算当前点与圆心的距离
int distance = sqrt(pow(x - centerX, 2) + pow(y - centerY, 2));
// 如果距离小于等于半径,则表示当前点在圆内
if (distance <= radius) {
printf("*");
} else {
printf(" "); // 圆外的点输出空格
}
}
printf("\n"); // 打印完一行后换行
}
return 0;
}
```
以上代码通过两层循环遍历每一个像素点,在每个像素点处判断该点是否在圆内,通过计算该点与圆心的距离来判断。若距离小于等于圆的半径,则该点在圆内,输出"*";否则输出空格。最后打印完一行后换行,形成一个圆形图案。
### 回答3:
下面是一个输出圆圈的C语言代码:
```c
#include <stdio.h>
#include <math.h>
// 圆的结构体定义
typedef struct {
float x; // 圆心的x坐标
float y; // 圆心的y坐标
float radius; // 圆的半径
} Circle;
// 输出圆心坐标和半径的函数
void printCircle(Circle c) {
printf("圆心坐标:(%.2f, %.2f)\n", c.x, c.y);
printf("圆的半径:%.2f\n", c.radius);
}
// 计算圆周长的函数
float calculateCircumference(Circle c) {
return 2 * M_PI * c.radius;
}
// 计算圆面积的函数
float calculateArea(Circle c) {
return M_PI * pow(c.radius, 2);
}
int main()
{
Circle myCircle; // 定义一个圆结构体变量
// 输入圆的信息
printf("请输入圆心的x坐标:");
scanf("%f", &myCircle.x);
printf("请输入圆心的y坐标:");
scanf("%f", &myCircle.y);
printf("请输入圆的半径:");
scanf("%f", &myCircle.radius);
// 输出圆的信息
printCircle(myCircle);
// 输出圆的周长
printf("圆的周长:%.2f\n", calculateCircumference(myCircle));
// 输出圆的面积
printf("圆的面积:%.2f\n", calculateArea(myCircle));
return 0;
}
```
这段代码中,定义了一个`Circle`结构体来表示圆,包括圆心的坐标和半径。`printCircle`函数用于输出圆的信息,`calculateCircumference`函数用于计算圆的周长,`calculateArea`函数用于计算圆的面积。在`main`函数中,首先定义一个`Circle`变量`myCircle`,然后通过输入语句获取用户输入的圆的信息,并输出圆的信息、周长和面积。
阅读全文
相关推荐
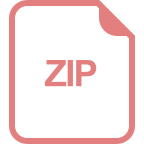
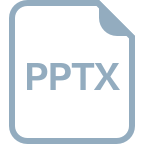
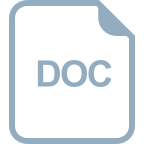
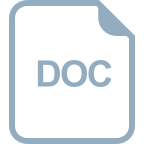
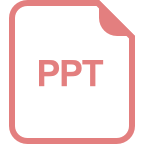
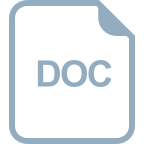
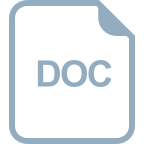
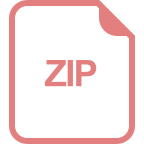
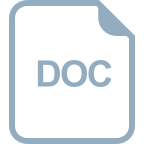
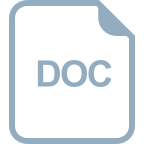
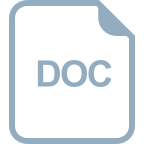
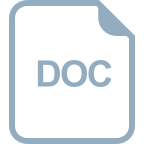
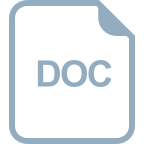
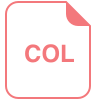

