1. 使用通用模型构建inception-lenet解决Mnist数字识别 python代码
时间: 2024-12-17 17:32:53 浏览: 3
在Python中,我们可以使用深度学习框架如TensorFlow或PyTorch构建一个结合Inception-v3和LeNet的思想的通用模型来解决MNIST手写数字识别任务。以下是一个简单的例子,我们将使用Keras库来构建这个模型:
首先,确保安装了必要的库:
```bash
pip install tensorflow numpy pandas keras
```
然后,编写代码:
```python
import tensorflow as tf
from tensorflow.keras.applications.inception_v3 import InceptionV3
from tensorflow.keras.layers import GlobalAveragePooling2D, Dense, Flatten, Input
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.datasets import mnist
from tensorflow.keras.utils import to_categorical
# 加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
x_train, x_test = x_train.reshape(-1, 28, 28, 1) / 255.0, x_test.reshape(-1, 28, 28, 1) / 255.0
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
# 准备InceptionV3模型作为基础特征提取器
base_model = InceptionV3(weights='imagenet', include_top=False, input_shape=(28, 28, 1))
# 添加全局平均池化层和全连接层
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(128, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# 创建新的模型,只训练顶部的几层
for layer in base_model.layers:
layer.trainable = False
# 定义完整模型
model = Model(inputs=base_model.input, outputs=predictions)
# 编译模型
model.compile(optimizer=Adam(lr=0.0001), loss='categorical_crossentropy', metrics=['accuracy'])
# 冻结基础模型训练,只训练新添加的部分
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test), batch_size=128)
# 解冻部分基础模型进行微调
for layer in base_model.layers[-5:]:
layer.trainable = True
# 继续训练整个模型
model.fit(x_train, y_train, epochs=10, initial_epoch=10, validation_data=(x_test, y_test), batch_size=128)
# 评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print(f'Test accuracy: {test_acc}')
阅读全文
相关推荐
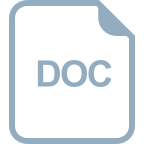
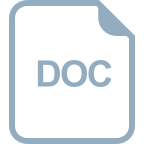
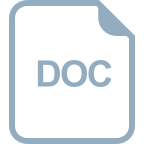

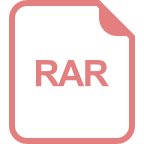
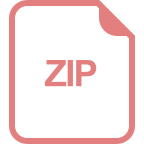
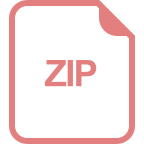
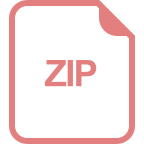
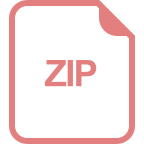
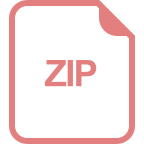
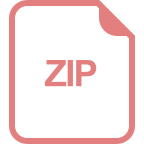
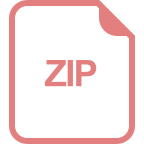
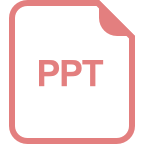
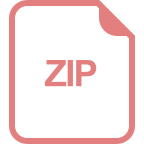
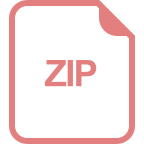
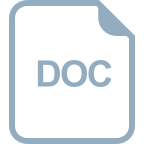
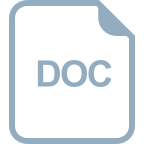
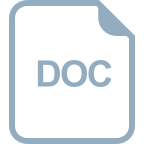