石头剪刀布c++语言
时间: 2024-09-10 16:18:14 浏览: 61
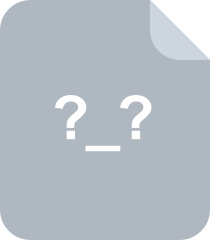
c++石头剪刀布游戏控制台实现
石头剪刀布是一个广泛流行的手势游戏,通常用于解决决策上的分歧。在C++语言中,我们可以通过简单的程序逻辑来模拟这个游戏。以下是一个简单的石头剪刀布游戏的C++代码示例:
```cpp
#include <iostream>
#include <stdlib.h> // 包含rand()和srand()
#include <time.h> // 包含time()
using namespace std;
// 函数声明
string getComputerChoice();
string getUserChoice();
void determineWinner(string userChoice, string computerChoice);
int main() {
string userChoice, computerChoice;
srand(time(NULL)); // 初始化随机数种子
computerChoice = getComputerChoice(); // 获取电脑的选择
userChoice = getUserChoice(); // 获取用户的选择
cout << "电脑选择: " << computerChoice << endl;
cout << "用户选择: " << userChoice << endl;
determineWinner(userChoice, computerChoice); // 判断胜负
return 0;
}
// 获取电脑的选择
string getComputerChoice() {
int choice = rand() % 3;
switch (choice) {
case 0: return "石头";
case 1: return "剪刀";
case 2: return "布";
default: return "";
}
}
// 获取用户的选择
string getUserChoice() {
string choice;
cout << "请选择:石头(0), 剪刀(1), 布(2): ";
cin >> choice;
return choice;
}
// 判断胜负
void determineWinner(string userChoice, string computerChoice) {
if (userChoice == computerChoice) {
cout << "平局!" << endl;
} else if ((userChoice == "石头" && computerChoice == "剪刀") ||
(userChoice == "剪刀" && computerChoice == "布") ||
(userChoice == "布" && computerChoice == "石头")) {
cout << "你赢了!" << endl;
} else {
cout << "你输了!" << endl;
}
}
```
这段代码中包含了一个简单的石头剪刀布游戏的逻辑。程序首先通过`getComputerChoice`函数随机生成电脑的选择,然后通过`getUserChoice`函数获取用户的选择,并通过`determineWinner`函数判断胜负结果。
阅读全文
相关推荐
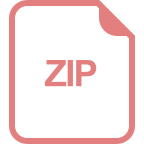
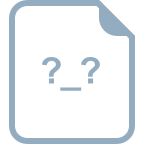
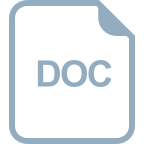
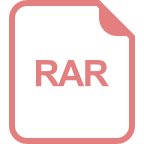
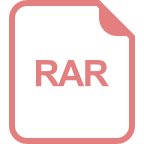
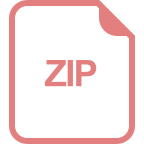
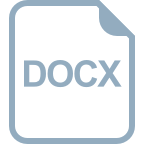
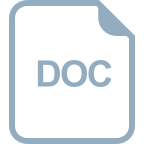
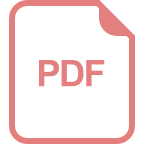
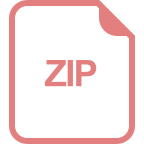
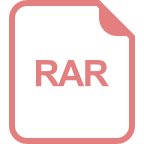
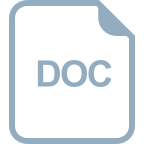
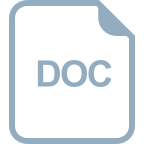
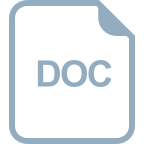
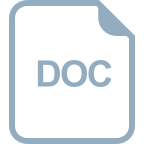
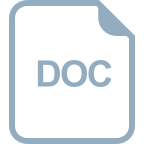

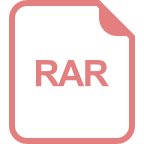
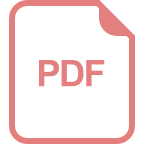