java生成pdf报表
时间: 2023-12-12 08:02:23 浏览: 91
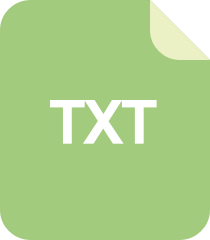
PDF报表例子~一个简单的例子
以下是使用Java生成PDF报表的步骤和示例代码:
1. 首先需要引入iText库,可以在Maven中添加以下依赖:
```xml
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
```
2. 创建一个PDF文档对象:
```java
Document document = new Document();
```
3. 创建一个PdfWriter对象,并将其与文档对象关联:
```java
PdfWriter.getInstance(document, new FileOutputStream("report.pdf"));
```
4. 打开文档对象:
```java
document.open();
```
5. 向文档对象中添加内容,例如段落、表格等:
```java
document.add(new Paragraph("Hello, World!"));
PdfPTable table = new PdfPTable(3);
table.addCell("Header 1");
table.addCell("Header 2");
table.addCell("Header 3");
table.addCell("1.1");
table.addCell("1.2");
table.addCell("1.3");
table.addCell("2.1");
table.addCell("2.2");
table.addCell("2.3");
document.add(table);
```
6. 关闭文档对象:
```java
document.close();
```
完整的示例代码如下:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class PdfReportGenerator {
public static void main(String[] args) {
try {
// 创建文档对象
Document document = new Document();
// 创建PdfWriter对象,并将其与文档对象关联
PdfWriter.getInstance(document, new FileOutputStream("report.pdf"));
// 打开文档对象
document.open();
// 向文档对象中添加内容
document.add(new Paragraph("Hello, World!"));
PdfPTable table = new PdfPTable(3);
table.addCell("Header 1");
table.addCell("Header 2");
table.addCell("Header 3");
table.addCell("1.1");
table.addCell("1.2");
table.addCell("1.3");
table.addCell("2.1");
table.addCell("2.2");
table.addCell("2.3");
document.add(table);
// 关闭文档对象
document.close();
} catch (FileNotFoundException | DocumentException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
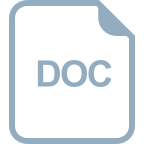
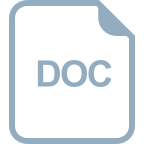
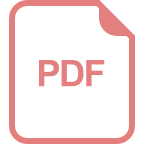
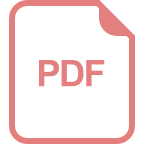
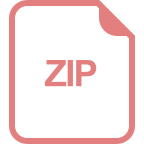
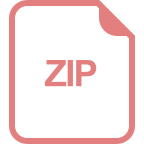
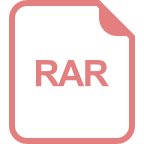
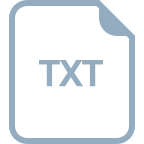
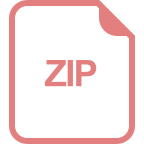
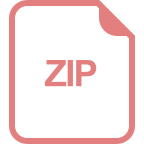
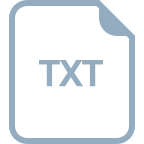
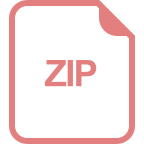
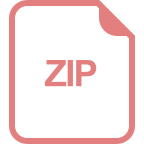
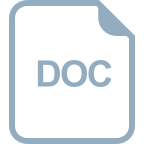
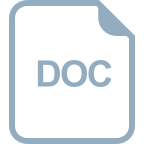
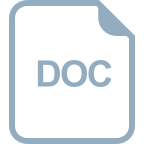