输入一个日期,格式如‘2024-09-24’,假设2月有28天,输出这一天是本年的第多少天
时间: 2024-09-24 15:31:15 浏览: 58
要计算给定日期是一年中的第几天,我们可以使用 Python 的 datetime 模块。首先,我们需要将字符串转换为 datetime 对象,然后利用 timedelta 函数来确定距离当年开始的天数。
以下是一个简单的示例代码:
```python
from datetime import datetime, timedelta
def days_in_year(date_string):
# 将输入的字符串格式化为 datetime 对象
date = datetime.strptime(date_string, '%Y-%m-%d')
# 创建一个从年初到该日期的 timedelta
since_epoch = date - datetime(date.year, 1, 1)
# 返回天数(包括开始那天)
return since_epoch.days + 1
# 测试一下
date_input = '2024-09-24'
day_of_year = days_in_year(date_input)
print(f'给定日期 {date_input} 是本年的第 {day_of_year} 天')
```
这段代码首先获取给定日期,然后计算从年初(1月1日)到这个日期有多少个完整的日子加上开始那天,从而得到一年中的总天数。
相关问题
编写一个c程序:输入一个日期,输出该日期是本年的第几天。(用if实现)需要考虑今年是闰年,输入形式:用横线分割的日期,格式为yyyy-mm-dd,输出形式:一个整数,表示该日期为本年的第几天
编写一个C语言程序,可以按照您的需求进行操作。首先,我们需要判断是否是闰年,并处理用户输入的日期。以下是一个基本的示例代码:
```c
#include <stdio.h>
#include <stdbool.h>
// 判断是否是闰年
bool is_leap_year(int year) {
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
return true;
}
else {
return false;
}
}
// 计算一年中某一天是第几天
int day_of_year(int year, int month, int day) {
// 年初天数
int days_in_month[] = {0, 31, 59, is_leap_year(year) ? 91 : 60, 89, 119, 149, 179, 209, 239, 269};
int total_days = 0;
for (int i = 0; i < month - 1; i++) {
total_days += days_in_month[i];
}
total_days += day;
return total_days;
}
int main() {
char date[11];
printf("请输入日期(格式 yyyy-mm-dd): ");
scanf("%s", date);
// 分割日期
char* parts[] = {date, NULL};
int year, month, day;
sscanf(parts[0], "%d-%d-%d", &year, &month, &day);
// 检查日期有效性
if(month <= 0 || month > 12 || day <= 0 || day > 31) {
printf("无效的日期格式\n");
return 1;
}
// 输出结果
int day_of_current_year = day_of_year(year, month, day);
printf("该日期是本年的第%d天。\n", day_of_current_year);
return 0;
}
```
在这个程序中,我们首先定义了一个`is_leap_year()`函数来检查是否是闰年。然后,在`day_of_year()`函数中计算给定日期是一年中的第几天。在`main()`函数里,我们获取用户的输入并进行相应的计算。
用c语言编程 【问题描述】输入一个日期,输出该日期是本年的第几天。 【提示】需要考虑本年是否为闰年 【输入形式】用横线分割的日期,格式为yyyy-mm-dd, 【输出形式】一个整数,表示该日期为本
在 C 语言中计算给定日期是本年的第几天,你需要处理以下几个步骤:
1. **判断是否为闰年**:
- 公元年份能被4整除但不能被100整除的是闰年;
- 能被400整除的也是闰年。
2. **将输入字符串转换为整数数组**:
使用 `sscanf` 函数解析输入的日期,将其拆分成年、月、日三个部分。
3. **计算天数**:
- 首先计算前一年的天数,包括平年和闰年的区别;
- 然后计算当前月份之前的所有月份天数(注意二月特殊情况);
- 最后加上当前月的日数。
4. **特殊处理2月**:
- 如果当前是2月,需区分平年和闰年,平年2月有28天,闰年2月有29天。
5. **输出结果**:
将累计的天数作为整数输出。
下面是一个简单的示例代码片段:
```c
#include <stdio.h>
#include <string.h>
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
if (month <= 2) {
year--;
month += 12;
}
switch(month) {
case 2:
return is_leap_year(year) ? 29 : 28;
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
int main() {
char date[11];
scanf("%s", date);
int year, month, day;
sscanf(date, "%d-%d-%d", &year, &month, &day);
// 判断闰年并计算总天数
int total_days = 365 + (is_leap_year(year) ? 1 : 0);
for (int i = 1; i < month; ++i) {
total_days += days_in_month(i, year);
}
total_days += day;
printf("该日期是%年%d月%d日,为本年的第%d天。\n", year, month, day, total_days);
return 0;
}
```
阅读全文
相关推荐
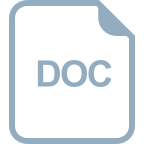
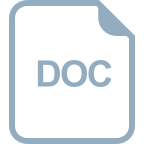
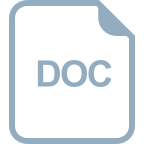













