springboot整合jwt跨域问题
时间: 2023-07-29 09:08:47 浏览: 60
Spring Boot整合JWT时,处理跨域问题可以采用以下几种方法:
1. 使用CORS(跨源资源共享)配置:在Spring Boot中,可以通过添加CORS配置来允许特定的源访问API。可以在`WebMvcConfigurer`配置类中添加`addCorsMappings`方法来实现。例如,允许所有源访问API可以使用以下配置:
```java
@Configuration
public class CorsConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*")
.allowedMethods("*")
.allowedHeaders("*")
.allowCredentials(true);
}
}
```
2. 使用Spring Security配置:如果你使用了Spring Security来保护API,并且已经配置了JWT认证,可以在`WebSecurityConfigurerAdapter`配置类中添加跨域配置。例如:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.cors().and().csrf().disable()
// 其他配置...
}
}
```
3. 使用过滤器处理跨域请求:可以创建一个自定义的过滤器来处理跨域请求。该过滤器可以在请求到达控制器之前添加跨域相关的响应头。例如:
```java
@Component
@Order(Ordered.HIGHEST_PRECEDENCE)
public class CorsFilter implements Filter {
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest request = (HttpServletRequest) req;
response.setHeader("Access-Control-Allow-Origin", "*");
response.setHeader("Access-Control-Allow-Methods", "POST, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Allow-Headers", "authorization, content-type");
response.setHeader("Access-Control-Max-Age", "3600");
if ("OPTIONS".equalsIgnoreCase(request.getMethod())) {
response.setStatus(HttpServletResponse.SC_OK);
} else {
chain.doFilter(req, res);
}
}
// 其他方法...
}
```
这些方法可以根据你的具体需求选择使用。通过配置CORS或Spring Security,或使用自定义过滤器,可以解决Spring Boot整合JWT时的跨域问题。
相关推荐
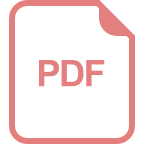
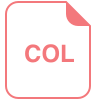
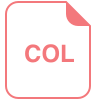
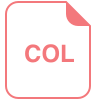
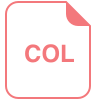









