python子类如何调用父类函数
时间: 2024-12-13 22:19:53 浏览: 11
在Python中,子类可以轻松地调用父类的函数,这是通过关键字`super()`来实现的。当你需要在子类的方法中访问并使用父类的同名方法时,可以这样做:
1. **继承上下文**:首先,确保你有一个派生类(子类),它从另一个类(父类)继承。
```python
class ParentClass:
def parent_function(self):
print("This is a function in the parent class.")
class ChildClass(ParentClass):
```
2. **调用父类函数**:在子类的方法内部,你可以使用`super().parent_function()`来调用父类的同名函数。例如,在`ChildClass`的构造函数`__init__`中:
```python
class ChildClass(ParentClass):
def __init__(self):
super().__init__() # 调用父类的初始化方法
self.parent_function() # 直接调用父类的函数
# 创建子类实例并显示结果
child = ChildClass()
```
这里,`super().__init__()`会自动调用父类的`__init__`方法,而`self.parent_function()`则是直接调用了`ParentClass`中的`parent_function`函数。
相关问题
python子类调用父类属性
在Python中,子类可以通过`super()`函数来调用父类的属性。具体的方法是在子类的初始化函数`__init__()`中调用`super().__init__()`来调用父类的初始化函数,从而获得父类的属性。例如:
```python
class Parent:
def __init__(self):
self.parent_property = "I am from Parent class"
class Child(Parent):
def __init__(self):
super().__init__()
self.child_property = "I am from Child class"
child = Child()
print(child.parent_property)
```
在这个例子中,子类`Child`继承了父类`Parent`,并且在子类的初始化函数中调用了父类的初始化函数,从而获得了父类的属性`parent_property`。最后,我们通过`print(child.parent_property)`打印出了子类的父类属性。
Python子类继承父类构造函数
可以通过super()函数调用父类构造函数。例如:
class ParentClass:
def __init__(self, name):
self.name = name
class ChildClass(ParentClass):
def __init__(self, name, age):
super().__init__(name)
self.age = age
在子类的构造函数中,可以调用super()函数来调用父类的构造函数,并传递相应的参数。这样,子类就可以继承父类的属性和方法,并且可以在此基础上增加自己的属性和方法。
阅读全文
相关推荐
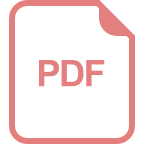
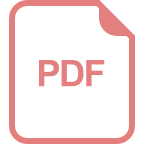
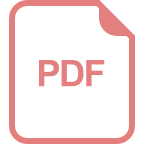
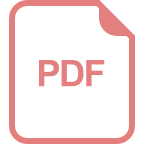
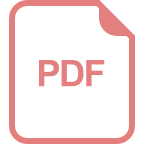
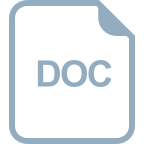









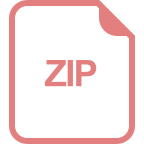