C++写个函数,实时获取windows的cpu使用率
时间: 2024-11-20 14:40:11 浏览: 62
在C++中,获取Windows系统的CPU使用率通常需要借助第三方库,如Boost或其他系统性能监控API。这里我将提供一个使用`boost::process`库的例子,它可以帮助我们读取性能计数器数据。请注意,你需要先安装并链接这个库。
```cpp
#include <boost/process.hpp>
#include <windows.h>
double get_cpu_usage() {
boost::process::winapi::performance_counters pc;
std::vector<boost::process::winapi::counter> counters = {
{"% Processor Time", "", "Processor"}
};
if (!pc.initialize()) {
throw std::runtime_error("Failed to initialize performance counters");
}
pc.select(counters);
double cpu_total = 0.0;
for (auto &counter : pc.counters()) {
DWORD value;
if (!counter.read(&value)) {
throw std::runtime_error("Failed to read counter value");
}
// Windows CPU usage is the total processor time divided by the number of logical processors
// Note that this can be an approximation due to the way Windows reports data
cpu_total += static_cast<double>(value) / GetLogicalProcessorCount();
}
return cpu_total / static_cast<double>(counters.size());
}
int main() {
try {
double usage = get_cpu_usage();
std::cout << "Current CPU usage: " << usage * 100 << "%" << std::endl;
} catch (const std::exception &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
阅读全文
相关推荐









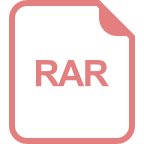
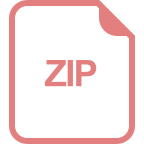






