Marshal.ReleaseComObject的使用方法,并给出代码示例
时间: 2024-11-23 10:50:50 浏览: 9
`Marshal.ReleaseComObject`是.NET Framework中用于释放COM对象的一个方法,它通常用于管理 COM 对象引用计数,防止内存泄漏。当你不再需要一个 COM 对象时,应该调用这个方法来减少对该对象的引用,以便垃圾回收器可以更有效地回收资源。
使用方法通常是这样的:
```csharp
using System.Runtime.InteropServices;
// 创建或获取到 COM 对象的实例
object comObject = ...;
try
{
// 使用 comObject
}
finally
{
// 当不再需要时释放
if (comObject isMarshalByRefObject)
{
Marshal.ReleaseComObject(comObject);
}
else
{
Marshal.FinalReleaseComObject(comObject);
}
}
```
这里需要注意的是,如果你的 `comObject` 实例实现了 `MarshalByRefObject` 接口,你应该使用 `Marshal.ReleaseComObject`;如果它不是,你应该使用 `Marshal.FinalReleaseComObject`。后者是 `Marshal.ReleaseComObject` 的最终版本,如果对象已经被正确地设置为不可见,它会立即释放资源。
相关问题
vb.net创建一个excel文件,并在单元格中插入图片,让图片四边刚好对齐单元格四边,给出一个完整vb.net代码示例
在VB.NET中,我们可以使用Microsoft.Office.Interop.Excel库来创建Excel文件并插入图片,确保图片四边与单元格对齐。这里是一个简单的示例:
```vb.net
Imports Microsoft.Office.Interop.Excel
Public Class ExcelInsertImage
Sub CreateExcelAndInsertImage()
' 创建一个新的Excel应用实例
Dim excelApp As New Application
Dim workbook As Workbook = excelApp.Workbooks.Add()
Dim worksheet As Worksheet = workbook.Sheets(1)
' 定义图片路径
Dim imagePath As String = "C:\path\to\your\image.jpg"
' 设置图片位置和大小,使其完全填充单元格
Dim cell As Range = worksheet.Cells(1, 1) ' 假设我们从A1开始
Dim pic As Picture = worksheet.Pictures.Insert(imagePath)
pic.Select()
pic.Left = cell.Left
pic.Top = cell.Top
pic.Width = cell.Width
pic.Height = cell.Height
' 使图片四周与单元格边缘对齐,这通常需要设置图片环绕模式
pic.ShapeStyle = msoShapeStyleNone ' 消除默认的轮廓样式
pic.WrapFormat.Alignment = XlWrapAlignment.xlCenterAcrossSelection
pic.WrapFormat.VAnchor = XlVAnchor.xlTop
' 关闭工作簿和保存文件
workbook.SaveAs("Output.xlsx")
workbook.Close()
excelApp.Quit()
' 清理资源
System.Runtime.InteropServices.Marshal.ReleaseComObject(worksheet)
System.Runtime.InteropServices.Marshal.ReleaseComObject(workbook)
System.Runtime.InteropServices.Marshal.ReleaseComObject(excelApp)
Console.WriteLine("图片已成功插入到Excel文件中.")
End Sub
End Class
```
在这个例子中,你需要替换`imagePath`变量为你实际图片的路径。运行这个代码后,会在指定的工作表A1位置插入图片,并保持图片与单元格四边对齐。
c# 如何使用 Microsoft.Office.Interop.Excel
以下是一个使用 C# 和 Microsoft.Office.Interop.Excel 操作 Excel 文件的简单示例:
1. 首先需要在项目中引用 Microsoft.Office.Interop.Excel 类库。
2. 在代码中创建 Excel 应用程序对象:
```csharp
using Excel = Microsoft.Office.Interop.Excel;
//...
Excel.Application excelApp = new Excel.Application();
```
3. 打开 Excel 文件,创建工作簿和工作表对象:
```csharp
Excel.Workbook workbook = excelApp.Workbooks.Open(@"path\to\file.xlsx");
Excel.Worksheet worksheet = workbook.Worksheets[1];
```
4. 读取或写入单元格数据:
```csharp
// 读取单元格数据
var value = worksheet.Cells[1, 1].Value;
// 写入单元格数据
worksheet.Cells[1, 1].Value = "Hello, world!";
```
5. 插入行列、合并单元格等操作:
```csharp
// 插入行
worksheet.Rows.Insert(2, 1);
// 插入列
worksheet.Columns.Insert(2, 1);
// 合并单元格
Excel.Range range = worksheet.Range[worksheet.Cells[1, 1], worksheet.Cells[2, 2]];
range.Merge();
```
6. 设置字体样式、单元格格式等:
```csharp
// 设置字体样式
Excel.Range range = worksheet.Cells[1, 1];
range.Font.Bold = true;
range.Font.Italic = true;
// 设置单元格格式
Excel.Range range = worksheet.Cells[1, 1];
range.NumberFormat = "0.00";
```
7. 保存并关闭 Excel 文件:
```csharp
workbook.Save();
workbook.Close();
```
需要注意的是,在最后要及时释放对象:
```csharp
System.Runtime.InteropServices.Marshal.ReleaseComObject(worksheet);
System.Runtime.InteropServices.Marshal.ReleaseComObject(workbook);
System.Runtime.InteropServices.Marshal.ReleaseComObject(excelApp);
```
以上就是一个简单的使用 C# 和 Microsoft.Office.Interop.Excel 操作 Excel 文件的示例。具体的操作可以根据需求进行修改和扩展。
阅读全文
相关推荐
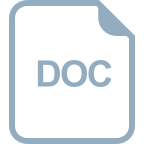
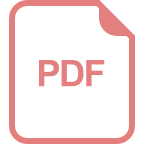
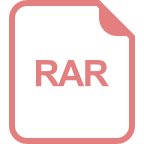













