android studio 设置下拉列表带图片
时间: 2023-08-09 10:06:56 浏览: 121
你可以通过自定义适配器来实现在 Android Studio 中设置下拉列表带图片。下面是一个简单的步骤:
1. 首先,在你的项目中创建一个新的适配器类,例如 `CustomAdapter`。
2. 在 `CustomAdapter` 类中,继承 `ArrayAdapter` 类,并重写 `getView()` 方法。
```java
public class CustomAdapter extends ArrayAdapter<String> {
private final Context context;
private final String[] values;
private final int[] images;
public CustomAdapter(Context context, String[] values, int[] images) {
super(context, R.layout.custom_spinner_item, values);
this.context = context;
this.values = values;
this.images = images;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View rowView = inflater.inflate(R.layout.custom_spinner_item, parent, false);
TextView textView = rowView.findViewById(R.id.text_view);
ImageView imageView = rowView.findViewById(R.id.image_view);
textView.setText(values[position]);
imageView.setImageResource(images[position]);
return rowView;
}
}
```
3. 创建一个自定义的布局文件 `custom_spinner_item.xml`,用于设置下拉列表每个选项的布局。该布局包含一个 `TextView` 和一个 `ImageView`。
```xml
<!-- custom_spinner_item.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<ImageView
android:id="@+id/image_view"
android:layout_width="24dp"
android:layout_height="24dp"
android:layout_marginRight="8dp" />
<TextView
android:id="@+id/text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
```
4. 在你的活动或片段中,初始化下拉列表并使用自定义适配器。
```java
String[] values = {"Option 1", "Option 2", "Option 3"};
int[] images = {R.drawable.image1, R.drawable.image2, R.drawable.image3};
Spinner spinner = findViewById(R.id.spinner);
CustomAdapter adapter = new CustomAdapter(this, values, images);
spinner.setAdapter(adapter);
```
确保将 `R.drawable.image1`、`R.drawable.image2`和`R.drawable.image3` 替换为你自己的图片资源。
这样,你就可以在 Android Studio 中设置下拉列表带图片了。希望对你有所帮助!
相关推荐
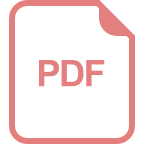
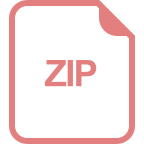
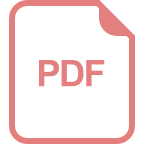














