linux中如何给进程上锁
时间: 2024-10-15 19:21:14 浏览: 23
在Linux中,为了保证进程间的同步和互斥访问,可以使用各种类型的锁机制。以下是几种常见的锁定方式:
1. **文件锁(flock)**:
使用`flock(2)`系统调用可以在一个打开的文件描述符上设置共享或独占锁。例如,`flockfile()`用于获取独占锁,`unlockfile()`释放锁。
```bash
#include <fcntl.h>
#include <unistd.h>
int lock_fd = open("lock_file", O_RDWR | O_CREAT);
if (lock_fd >= 0) {
flock(lock_fd, LOCK_EX); // 独占锁
... // 执行操作
unlock(lock_fd);
close(lock_fd);
}
```
2. **信号量(semaphore)**:
使用`sem_init()`创建一个信号量,`sem_wait()`、`sem_post()`等函数进行等待和释放。 semaphore提供了更精细的控制,如计数信号量。
```c
#include <semaphore.h>
sem_t sem;
if (sem_init(&sem, 0, 1)) { /* 初始化为1 */
sem_wait(&sem); // 等待
... // 操作
sem_post(&sem); // 释放
}
sem_destroy(&sem); // 销毁信号量
```
3. **互斥锁(mutex)**:
Linux提供了一些库如`pthread_mutex`,用于在多线程环境下互斥访问资源。
```c
#include <pthread.h>
pthread_mutex_t mtx;
pthread_mutexattr_t attr;
if (pthread_mutexattr_init(&attr) == 0) {
pthread_mutexattr_settype(&attr, PTHREAD_MUTEX_RECURSIVE); // 设置递归锁
if (pthread_mutex_init(&mtx, &attr) == 0) {
pthread_mutex_lock(&mtx); // 上锁
... // 操作
pthread_mutex_unlock(&mtx); // 解锁
}
pthread_mutexattr_destroy(&attr);
}
pthread_mutex_destroy(&mtx);
```
4. **条件变量(condvar)**:
结合信号量或互斥锁,`pthread_cond_wait()`和`pthread_cond_signal()`可以实现在满足特定条件后唤醒等待的进程。
在使用以上锁机制时,需要确保正确处理锁的获取、释放以及异常情况,以避免死锁和资源竞争等问题。
阅读全文
相关推荐




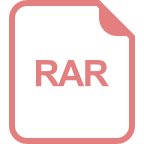






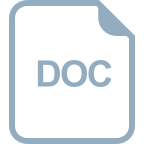



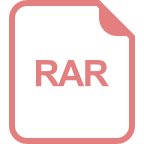

