Enter+at+least+4+floating+point+numbers.+After+removing+the+maximum+value,+calculate+their+average+s
时间: 2023-12-14 22:33:06 浏览: 52
以下是Python代码,用于输入至少4个浮点数,删除最大值并计算其平均值:
```python
# 输入至少4个浮点数
nums = []
while len(nums) < 4:
num = input("请输入一个浮点数:")
try:
num = float(num)
nums.append(num)
except:
print("输入无效,请重新输入!")
# 删除最大值并计算平均值
max_num = max(nums)
nums.remove(max_num)
avg = sum(nums) / len(nums)
print("删除最大值后的平均值为:", avg)
```
相关问题
keil+floating+point+support+not+loaded
根据您提供的引用内容,"floating point support not loaded"是一个错误消息,通常在使用Keil编译器时出现。这个错误消息表示编译器无法正确加载浮点数支持库。
解决这个问题的方法是在Keil编译器中启用浮点数支持。您可以按照以下步骤进行操作:
1. 打开Keil编译器。
2. 在菜单栏中选择"Project"(项目)。
3. 选择"Options for Target"(目标选项)。
4. 在弹出的对话框中,选择"Target"(目标)选项卡。
5. 在"Floating-point hardware"(浮点硬件)下拉菜单中,选择适合您的目标硬件的选项。如果您的硬件不支持浮点数运算,可以选择"None"(无)选项。
6. 单击"OK"(确定)按钮保存更改。
7. 重新编译您的程序,应该不再出现"floating point support not loaded"错误。
请注意,具体的步骤可能会因Keil编译器的版本而有所不同。以上步骤仅供参考,请根据您使用的具体版本进行操作。
floating-point+does+not+fit+in+required+integral+type
浮点数不适合所需的整数类型是指在将浮点数赋值给整数类型时,可能会发生溢出或精度丢失的情况。这是因为浮点数和整数类型内部表示和表示范围上有所同。
浮点数是用于表示带有数部分的数字的数据类型,而整数类型只能表示没有小数部分的数字。当将浮点数赋值给一个整数类型,编译器会尝试将浮点的值转换为整数类型的值。然而,由于浮点数的表示范围比整数类型大,或者浮点数的小数分无法准确地表示为整数,可能会导致溢出或精度丢失。
例如,将一个很大的浮点数赋值给一个较小的整数类型时,可能会发生出。另外,浮点数的小数部分可能无法准确地表示为整数,这可能导致精度丢失。
为了解决这个问题,可以使用类型转换操作符或者四舍五入等方法来处理浮点数和整数类型之间的转换。
相关推荐
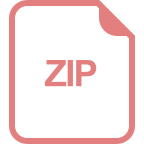












