qt中清除qcustomplot上的所有波形图的函数代码
时间: 2024-10-21 12:02:44 浏览: 43
在Qt的QCustomPlot库中,如果你想要清除所有的波形图,你可以使用`clearPlottable()`函数。这个函数需要传入对应的`QCPItem`,在这里通常是`QCPGraph`对象,它是绘制图形的基本元素。下面是一个简单的示例代码:
```cpp
QCustomPlot *customPlot = new QCustomPlot(parent); // 初始化你的QCustomPlot实例
// 添加并显示波形图
QCPGraph *graph = customPlot->addGraph();
graph->setData(xData, yData); // xData 和 yData 是你的数据
// 清除所有波形图(假设你只有一个)
for (int i = 0; i < customPlot->graphs().count(); ++i) {
if (auto graphToRemove = dynamic_cast<QCPGraph*>(customPlot->itemAt(i))) {
graphToRemove->clearPlottable(); // 移除波形图
}
}
```
在这个例子中,我们遍历了`graphs()`迭代器来检查每个QCPItem是否是QCPGraph类型的,如果是,则调用`clearPlottable()`。注意,你需要确保已经创建了对应的QCPGraph实例,并将其添加到QCustomPlot中。
相关问题
qt按钮清除qcustomplot上的所有波形图的代码
在Qt中,特别是使用QCustomPlot库绘制图形时,如果你想创建一个按钮来清除QCustomPlot上的所有波形图,你可以这样做:
首先,你需要包含必要的头文件,并且需要有QCustomPlot对象的实例,以及一个QPushButton对象。这里是一个简单的例子:
```cpp
#include <QApplication>
#include <QMainWindow>
#include "qcustomplot.h"
#include "ui_mainwindow.h"
class ClearButton : public QPushButton
{
public:
explicit ClearButton(QWidget *parent = nullptr) : QPushButton("清除波形", parent) {
connect(this, &ClearButton::clicked, qcp, &QCustomPlot::clearPlots);
}
private:
QCustomPlot *qcp;
};
int main(int argc, char *argv[]) {
QApplication a(argc, argv);
QMainWindow window;
Ui_MainWindow ui;
ui.setupUi(&window);
// 初始化QCustomPlot
QCustomPlot *customPlot = new QCustomPlot(&window);
customPlot->addGraph(); // 添加默认的波形图
// 创建并添加清除按钮
ClearButton clearButton;
clearButton.resize(100, 50);
clearButton.move(window.width() - clearButton.width(), 10); // 将按钮放在窗口底部
window.setCentralWidget(customPlot);
window.show();
return a.exec();
}
```
在这个示例中,`ClearButton`继承自QPushButton,当点击按钮时,会连接到`QCustomPlot`的`clearPlots()`方法,该方法用于清除所有的波形图。
qcustomplot绘制实时波形图
QCustomPlot 是一款功能强大的 Qt 绘图库,它支持多种图表类型,并且具有丰富的交互功能和自定义能力。
要在 QCustomPlot 中实现实时波形图,可以按照以下步骤进行:
1. 创建一个 QCustomPlot 对象,并添加一个 QCPGraph 对象作为波形图的曲线。
```cpp
QCustomPlot *customPlot = new QCustomPlot(this);
QCPGraph *graph = customPlot->addGraph();
```
2. 设置波形图的样式,包括线条颜色、宽度、样式等。
```cpp
graph->setPen(QPen(Qt::blue));
graph->setLineStyle(QCPGraph::lsLine);
graph->setAntialiased(true);
```
3. 在每次需要更新波形图时,向 QCPGraph 中添加新的数据点,并重新绘制图形。
```cpp
double time = QDateTime::currentDateTime().toMSecsSinceEpoch()/1000.0; // 获取当前时间戳
double value = ...; // 获取需要绘制的数据值
graph->addData(time, value); // 添加新的数据点
customPlot->rescaleAxes(); // 自适应缩放坐标轴
customPlot->replot(); // 重新绘制图形
```
4. 在实时绘制过程中,为了不影响绘图性能,可以设置 QCustomPlot 对象的自动重绘模式为“不自动重绘”。
```cpp
customPlot->setNoAntialiasingOnDrag(true);
customPlot->setInteractions(QCP::iRangeDrag | QCP::iRangeZoom | QCP::iSelectPlottables);
customPlot->setAntialiasedElements(QCP::aeNone);
customPlot->setNotAntialiasedElements(QCP::aeAll);
customPlot->setAutoAddPlottableToLegend(false);
customPlot->setAutoMargin(true);
customPlot->setAutoSubTicks(true);
customPlot->setAutoTickStep(true);
customPlot->setMultiSelectModifier(Qt::ControlModifier);
customPlot->setNoAntialiasingOnDrag(true);
customPlot->setOpenGl(true);
customPlot->setPlottingHint(QCP::phFastPolylines, true);
customPlot->setInteractions(QCP::iRangeDrag | QCP::iRangeZoom | QCP::iSelectPlottables);
```
以上是使用 QCustomPlot 绘制实时波形图的基本步骤,实际应用中,还可以根据需要进行细节调整和性能优化。
阅读全文
相关推荐
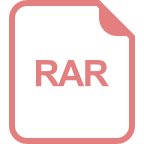
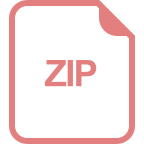
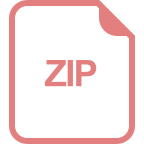
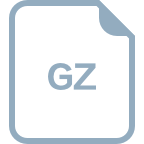
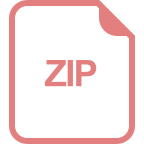
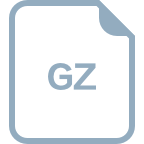
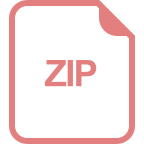

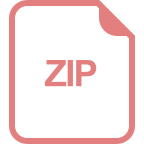
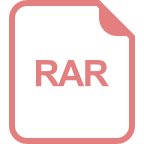
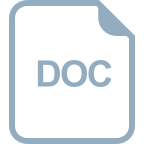
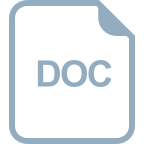


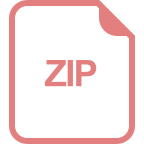
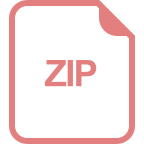
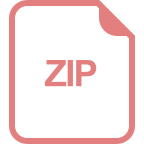