springboot使用http调用星火大模型接口
时间: 2024-09-15 17:04:13 浏览: 61
Spring Boot 是一个轻量级的 Java 框架,用于简化初始搭建和开发过程。如果你想利用 Spring Boot 来调用外部的 HTTP 接口,比如星火大模型的 API,你可以按照以下步骤操作:
1. **添加依赖**:首先,在你的 Maven 或者 Gradle 项目中添加对 HTTP Client 的依赖,如 `org.springframework.boot:spring-boot-starter-web` 对于 RESTful 调用,或者 `com.squareup.okhttp3:okhttp` 或 `ioktor:ktor-client-java` 等库。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Gradle (using Spring Boot starters) -->
implementation 'org.springframework.boot:spring-boot-starter-web'
```
2. **创建配置**:如果你使用的是 Spring Boot,可以在 `application.properties` 或 `application.yml` 中配置默认的 HTTP 服务连接超时、请求头等。
```yaml
# application.yml
http:
client:
timeout: 5s
connect-timeout: 3s
```
3. **编写客户端代码**:在需要调用 API 的地方,可以使用 `RestTemplate` 或 `WebClient`(Spring 5+推荐)来发起请求。
```java
import org.springframework.web.reactive.function.client.WebClient;
// 使用 RestTemplate
@Autowired
private RestTemplate restTemplate;
public String callModelApi(String apiUrl) {
ResponseEntity<String> response = restTemplate.getForEntity(apiUrl, String.class);
return response.getBody();
}
// 使用 WebClient ( reactive 方式)
@Autowired
private WebClient webClient;
public Mono<String> callModelApiReactive(String apiUrl) {
return webClient.get().uri(apiUrl).retrieve().bodyToMono(String.class);
}
```
4. **处理错误和异常**:别忘了处理可能出现的网络错误、API 错误等,并适当地将响应状态码、错误信息返回给用户或日志系统。
5. **测试与部署**:在单元测试或者集成测试中验证 API 调用是否正常,然后部署到生产环境。
阅读全文
相关推荐
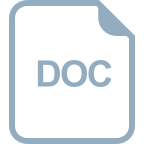
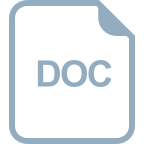
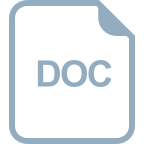
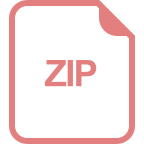
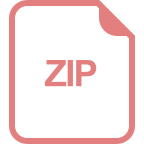
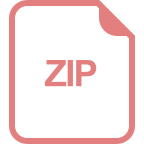
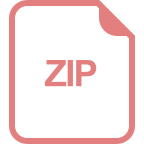
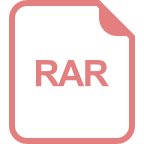
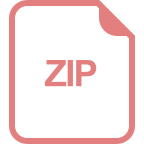
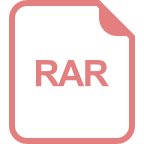
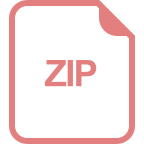
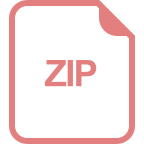
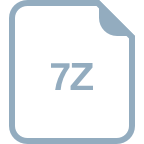
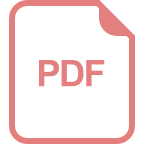
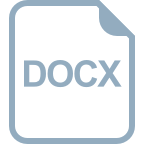
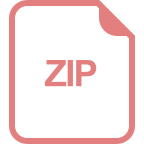
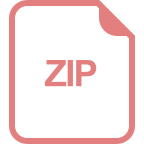
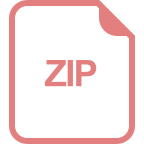