JAVA循环队列实现
时间: 2023-09-03 13:12:44 浏览: 105
循环队列是一种经典的数据结构,在Java中可以使用数组来实现。下面是一个简单的Java循环队列的实现示例:
```java
public class CircularQueue {
private int[] queue;
private int front; // 队头指针
private int rear; // 队尾指针
private int size; // 队列的当前元素个数
public CircularQueue(int capacity) {
queue = new int[capacity];
front = 0;
rear = 0;
size = 0;
}
public boolean isEmpty() {
return size == 0;
}
public boolean isFull() {
return size == queue.length;
}
public void enqueue(int item) {
if (isFull()) {
throw new IllegalStateException("队列已满");
}
queue[rear] = item;
rear = (rear + 1) % queue.length; // 指针移动到下一个位置,考虑循环
size++;
}
public int dequeue() {
if (isEmpty()) {
throw new IllegalStateException("队列为空");
}
int item = queue[front];
front = (front + 1) % queue.length; // 指针移动到下一个位置,考虑循环
size--;
return item;
}
public int peek() {
if (isEmpty()) {
throw new IllegalStateException("队列为空");
}
return queue[front];
}
public int size() {
return size;
}
}
```
上述代码中,`enqueue` 方法用于入队,`dequeue` 方法用于出队,`peek` 方法用于获取队头元素,`size` 方法用于获取队列当前元素个数。
请注意,队列的大小是固定的,即创建队列时需要指定容量。在入队和出队操作时,使用了取模运算来实现指针的循环移动。
阅读全文
相关推荐
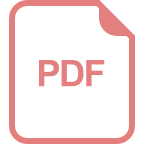
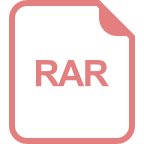
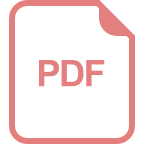
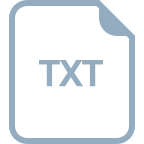
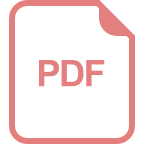
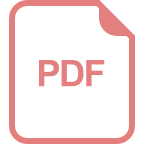
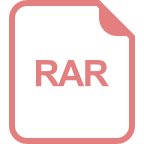
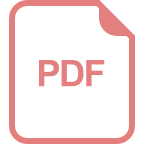
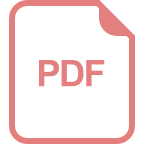
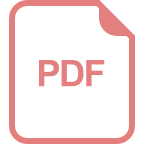
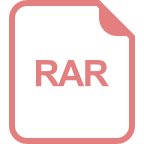
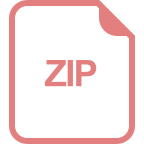
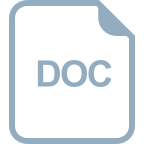
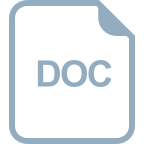
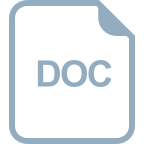
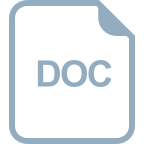