用vc++6.0写一个班上有30名学生,每个学生的数据作为一个记录,每个记录包括学号、姓名、三门课程的成绩和三门课程平均成绩。从键盘输入学生的学号、姓名及三门课。的代码 (2)将程序改为使用链表实现。
时间: 2024-10-16 09:02:46 浏览: 26
首先,我们来讲解如何在VC++6.0中使用结构体和数组来存储学生信息。假设有一个名为`Student`的结构体,包含学号、姓名和成绩等字段:
```cpp
#include <iostream>
using namespace std;
struct Student {
int id;
string name;
double score[3]; // 存储三门课程的成绩
};
int main() {
const int num_students = 30;
Student students[num_students];
for (int i = 0; i < num_students; ++i) {
cout << "请输入第" << (i + 1) << "位学生的学号:";
cin >> students[i].id;
cout << "请输入第" << (i + 1) << "位学生姓名:";
cin >> students[i].name;
for (int j = 0; j < 3; ++j) {
cout << "请输入第" << (j + 1) << "门课程的成绩:";
cin >> students[i].score[j];
}
// 计算平均成绩并输出
double average = (students[i].score[0] + students[i].score[1] + students[i].score[2]) / 3.0;
cout << "第" << i+1 << "位学生的平均成绩是:" << average << endl;
}
return 0;
}
```
接下来,我们将这个程序转换成使用链表来存储学生信息。首先创建一个`StudentNode`结构体作为链表节点,并包含指向下一位学生的指针以及学生信息:
```cpp
#include <iostream>
#include <string>
using namespace std;
struct StudentNode {
int id;
string name;
double score[3];
StudentNode* next; // 指向下一个节点
};
class StudentList {
private:
StudentNode* head;
public:
void insertStudent(int id, string name) {
StudentNode* newNode = new StudentNode();
newNode->id = id;
newNode->name = name;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
} else {
StudentNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 其他方法,如计算平均成绩和遍历链表...
};
int main() {
StudentList studentList;
// 使用insertStudent方法添加学生信息...
// 计算平均成绩、打印列表等操作
return 0;
}
```
阅读全文
相关推荐
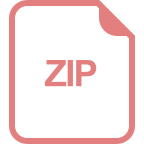
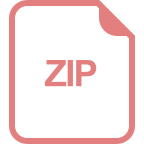
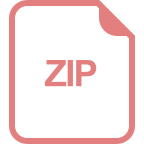
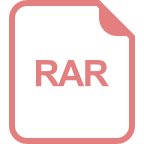
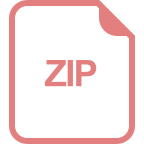
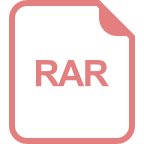
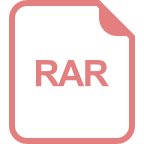
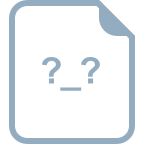
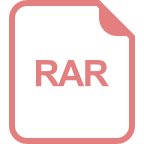
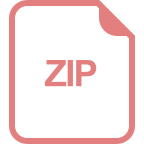
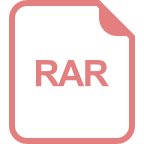
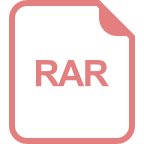
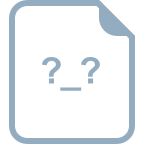
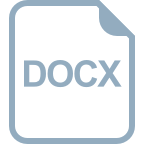
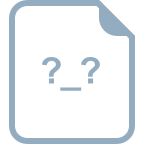